Hello, I am a Python programming enthusiast passionate about sharing programming knowledge. Today, let's discuss the important topic of Python object-oriented programming. Object-oriented programming is one of the core concepts in Python programming. Once you master it, you'll be able to write more elegant and maintainable code. Let's explore this topic step by step!
From Blueprint to Physical Object
Do you know how construction workers build a building? They first need a blueprint that details how each part of the building should be constructed. A class is like this blueprint, describing the attributes and behaviors of objects. An object is the physical entity created based on this blueprint.
For example, let's define a Dog
class that includes some attributes and methods of dogs:
class Dog:
def __init__(self, name, breed):
self.name = name
self.breed = breed
def bark(self):
print(f"{self.name} is barking!")
In the code above, Dog
is a class with attributes name
and breed
, and a method bark()
. Now we can create dog objects based on this class:
buddy = Dog("Buddy", "Golden Retriever")
buddy.bark() # Buddy is barking!
We instantiated a Golden Retriever dog object named buddy
, which has its own name and breed attributes, and can call the bark()
method. Isn't it amazing? A class is like a mold from which we can create countless objects.
Code Genetic Inheritance
In real life, a child inherits certain characteristics from their parents. Similarly, in programming, a subclass can inherit attributes and methods from its parent class. This process is called inheritance.
Suppose we also want to define a Cat
class that shares some common attributes and methods with the Dog
class, such as having a name
attribute and being able to make_sound()
. We can first define a parent class Pet
:
class Pet:
def __init__(self, name):
self.name = name
def make_sound(self):
pass # Subclasses need to implement this method
Then both Dog
and Cat
classes inherit from the Pet
class:
class Dog(Pet):
def __init__(self, name, breed):
super().__init__(name) # Call parent class constructor
self.breed = breed
def make_sound(self):
print(f"{self.name} is barking!")
class Cat(Pet):
def make_sound(self):
print(f"{self.name} is meowing!")
Now both Dog
and Cat
objects can use the make_sound()
method:
buddy = Dog("Buddy", "Golden Retriever")
buddy.make_sound() # Buddy is barking!
kitty = Cat("Kitty")
kitty.make_sound() # Kitty is meowing!
Through inheritance, we can reuse code and avoid rewriting the same logic. This improves code maintainability and extensibility.
Adding and Subtracting Objects
In Python, we can even overload operators to allow objects to support mathematical operations like addition and subtraction. This requires the use of some "magic methods", such as __add__
for overloading the +
operator.
Suppose we have a Vector
class to represent two-dimensional vectors, and we want to be able to use the +
operator directly to add two vector objects. We can define the __add__
method like this:
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
x = self.x + other.x
y = self.y + other.y
return Vector(x, y)
v1 = Vector(1, 2)
v2 = Vector(3, 4)
v3 = v1 + v2 # Equivalent to v3 = v1.__add__(v2)
print(v3.x, v3.y) # Outputs 4 6
By overloading the __add__
method, we've made it possible to add two Vector
objects directly using the +
operator. This not only makes the code more concise and readable but also increases extensibility, as we can add more "operator" behaviors to the Vector
class.
The Art of Encapsulation
Have you ever encountered a situation where you only want to access and modify an object's attributes internally, without allowing external code to change them arbitrarily? This is where encapsulation comes in.
In Python, we can use the @property
decorator to implement attribute encapsulation. It allows us to define getter and setter methods to control read and write access to attributes.
class BankAccount:
def __init__(self, balance=0):
self._balance = balance # Underscore prefix indicates internal attribute
@property
def balance(self):
return self._balance
@balance.setter
def balance(self, new_balance):
if new_balance < 0:
print("Balance cannot be negative")
else:
self._balance = new_balance
account = BankAccount(1000)
print(account.balance) # 1000
account.balance = 2000 # Normal modification
account.balance = -500 # Balance cannot be negative
In the code above, we've encapsulated the _balance
attribute, which can only be read and written through the getter and setter methods of the balance
property. This way, we can add some restrictions in the setter method, such as not allowing negative balances.
Encapsulation not only improves code security but also enhances code maintainability and extensibility. We can modify the implementation details of internal attributes without changing external code.
Summary and Reflection
Today, we've learned the basics of Python object-oriented programming, including class and object definitions, inheritance, operator overloading, and attribute encapsulation. With these concepts mastered, you'll be able to write more elegant and reusable Python code.
However, object-oriented programming is far more than just these concepts. It also includes advanced concepts like abstraction, polymorphism, and design patterns. I'll continue to explore these topics in my next article.
Finally, I'd like you to ponder a question: In actual development, how should we balance between over-design and sufficient design? Over-design can lead to overly complex code, while insufficient design can affect code maintainability. Do you have any suggestions? Feel free to leave your thoughts in the comments section.
The journey of programming is long and challenging. Let's continue to learn, share, and progress together on this path!
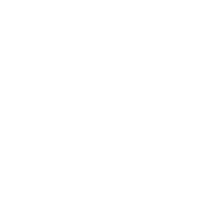
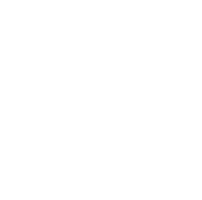