Hello, dear Python learner! Today, we embark on a marvelous journey to explore the exciting world of Python Object-Oriented Programming (OOP). As a Python programming blogger, I’m often asked, "What exactly is Object-Oriented Programming? Why should we learn it?"
To be honest, I was baffled when I first encountered OOP. But with deeper learning and practice, I found that OOP not only makes code more elegant and maintainable, but also helps us think about problems in a new way. Today, let's unveil the mystery of OOP and see how it changes our programming approach!
Introduction
Remember when you first started programming? Back then, we might only write simple functions, separating data and the code that manipulates it. But as projects get more complex, this approach quickly becomes hard to manage. That’s when OOP comes into play!
The core idea of OOP is to package data and the functions that operate on it into "objects." You can think of objects as entities in the real world, like a cat, a car, or a student. Each object has its own attributes (data) and methods (functions).
Let's start with a simple example:
class Cat:
def __init__(self, name, age):
self.name = name
self.age = age
def meow(self):
print(f"{self.name}: Meow!")
my_cat = Cat("Whiskers", 3)
my_cat.meow() # Output: Whiskers: Meow!
See that? We created a Cat
class with name
and age
attributes, and a meow
method. Then we used this class to create a cat object named Whiskers. Isn’t it intuitive?
In-Depth
The Magic of Encapsulation
One important feature of OOP is encapsulation. Encapsulation is like putting a protective layer around an object, exposing only necessary interfaces and hiding internal details. This not only protects data from being modified arbitrarily but also makes code more modular and maintainable.
Here’s an example:
class BankAccount:
def __init__(self, balance):
self.__balance = balance # Private attribute
def deposit(self, amount):
if amount > 0:
self.__balance += amount
return True
return False
def withdraw(self, amount):
if 0 < amount <= self.__balance:
self.__balance -= amount
return True
return False
def get_balance(self):
return self.__balance
account = BankAccount(1000)
print(account.get_balance()) # Output: 1000
account.deposit(500)
print(account.get_balance()) # Output: 1500
In this example, we set the balance __balance
as a private attribute, inaccessible directly from outside. To deposit or withdraw money, you must use the deposit
and withdraw
methods. This ensures the safety and consistency of the balance.
Have you ever thought about what would happen without encapsulation? Imagine if anyone could directly modify your bank account balance; that would be terrifying!
The Power of Inheritance
Inheritance is another powerful feature of OOP. It allows us to create new classes based on existing ones. The new class can inherit attributes and methods from the parent class and add its own features. This greatly improves code reusability.
Here’s an example:
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
pass
class Dog(Animal):
def speak(self):
return f"{self.name} says Woof!"
class Cat(Animal):
def speak(self):
return f"{self.name} says Meow!"
dog = Dog("Buddy")
cat = Cat("Whiskers")
print(dog.speak()) # Output: Buddy says Woof!
print(cat.speak()) # Output: Whiskers says Meow!
See that? Both Dog
and Cat
classes inherit from the Animal
class. They have the name
attribute and the speak
method. But each class has its unique speak
implementation. That’s the magic of inheritance!
I remember when I first understood inheritance, it felt like opening a door to a new world. Suddenly, the code became so elegant and logical. Have you had a similar experience?
The Wonder of Polymorphism
Polymorphism is another important OOP feature. It allows us to handle different types of objects in a uniform way. This not only makes the code more flexible but also greatly enhances its scalability.
Let’s see an example:
def animal_concert(animals):
for animal in animals:
print(animal.speak())
dog = Dog("Buddy")
cat = Cat("Whiskers")
parrot = Parrot("Polly") # Suppose we have a Parrot class
animal_concert([dog, cat, parrot])
In this example, the animal_concert
function doesn’t need to care about what type of animal is passed in, as long as they all have a speak
method. That’s the power of polymorphism!
I once used polymorphism in a large project to handle different types of user input. It made the code exceptionally clear and easy to extend. Can you think of how to apply polymorphism in your projects?
Advanced
The Magic of Magic Methods
Python has some special methods with double underscores before and after their names, like __init__
, __str__
, etc. These are called magic methods or dunder methods. They allow us to customize the behavior of a class, making its use more Pythonic.
Here’s an example:
class Book:
def __init__(self, title, author, pages):
self.title = title
self.author = author
self.pages = pages
def __str__(self):
return f"{self.title} by {self.author}"
def __len__(self):
return self.pages
book = Book("Python Tricks", "Dan Bader", 301)
print(book) # Output: Python Tricks by Dan Bader
print(len(book)) # Output: 301
See that? By defining the __str__
method, we can customize the string representation of an object. The __len__
method lets us use the len()
function to get the book's number of pages.
These magic methods are like giving classes magical powers, allowing them to seamlessly integrate with Python's built-in functions and operations. Can you think of other useful magic methods?
The Charm of Property Decorators
The @property
decorator in Python is a powerful tool. It allows us to call methods as if they were attributes, which not only makes the code more elegant but also adds extra logic without changing the class interface.
Here’s an example:
class Circle:
def __init__(self, radius):
self._radius = radius
@property
def radius(self):
return self._radius
@radius.setter
def radius(self, value):
if value < 0:
raise ValueError("Radius cannot be negative")
self._radius = value
@property
def area(self):
return 3.14 * self._radius ** 2
circle = Circle(5)
print(circle.radius) # Output: 5
print(circle.area) # Output: 78.5
circle.radius = 10
print(circle.area) # Output: 314.0
circle.radius = -1 # Raises ValueError
In this example, we use the @property
decorator to turn radius
and area
methods into properties. This way, we can use them like attributes while retaining the flexibility of methods.
I particularly love using property decorators because they make the code both concise and powerful. What do you think?
Advanced Applications of Metaclasses
Metaclasses are a relatively advanced concept in Python, allowing us to control the creation process of classes. While they might not be needed in everyday programming, understanding metaclasses can help us gain deeper insight into Python’s class mechanism.
Here’s a simple example:
class SingletonMeta(type):
_instances = {}
def __call__(cls, *args, **kwargs):
if cls not in cls._instances:
cls._instances[cls] = super().__call__(*args, **kwargs)
return cls._instances[cls]
class Singleton(metaclass=SingletonMeta):
def __init__(self):
self.value = None
s1 = Singleton()
s2 = Singleton()
print(s1 is s2) # Output: True
In this example, we use a metaclass to implement the singleton pattern. No matter how many times we create an instance of the Singleton
class, we get the same object.
While powerful, metaclasses can also be easily misused. Remember, simplicity is often best. Use metaclasses only when truly needed. Can you think of other scenarios to use metaclasses?
Practical Application
After all this theory, let’s see how to apply OOP in a real project!
Suppose we’re developing a simple book management system. We might define these classes:
class Book:
def __init__(self, title, author, isbn):
self.title = title
self.author = author
self.isbn = isbn
self.is_borrowed = False
def __str__(self):
return f"{self.title} by {self.author}"
class Member:
def __init__(self, name, member_id):
self.name = name
self.member_id = member_id
self.borrowed_books = []
def borrow_book(self, book):
if not book.is_borrowed:
self.borrowed_books.append(book)
book.is_borrowed = True
return True
return False
def return_book(self, book):
if book in self.borrowed_books:
self.borrowed_books.remove(book)
book.is_borrowed = False
return True
return False
class Library:
def __init__(self):
self.books = []
self.members = []
def add_book(self, book):
self.books.append(book)
def add_member(self, member):
self.members.append(member)
def find_book(self, isbn):
for book in self.books:
if book.isbn == isbn:
return book
return None
library = Library()
book1 = Book("Python Tricks", "Dan Bader", "1234567890")
book2 = Book("Fluent Python", "Luciano Ramalho", "0987654321")
library.add_book(book1)
library.add_book(book2)
member = Member("Alice", "M001")
library.add_member(member)
if member.borrow_book(book1):
print(f"{member.name} borrowed {book1}")
if library.find_book("0987654321"):
print("Book found!")
else:
print("Book not found.")
See that? By using OOP, we can naturally simulate real-world entities and their relationships. The Book
, Member
, and Library
classes each encapsulate related data and operations, making the code structure clear and easy to understand and maintain.
In real development, we might need to add more features, such as handling overdue items or calculating fines. But with this foundational structure, adding new features becomes very easy.
Can you think of how to improve this system? Maybe by adding a Librarian
class to handle some management tasks? Or using inheritance to handle different types of books?
Conclusion
Our journey with OOP comes to an end here. We started with basic concepts, gradually delved into some advanced features, and finally saw a practical example. I hope this journey has given you a deeper understanding of OOP!
Remember, OOP is not just a programming paradigm but a way of thinking about problems. It encourages us to view problems from the perspective of objects, which often leads to new insights and solutions.
Of course, OOP is not a panacea. In some cases, functional programming or procedural programming might be more appropriate. As a good programmer, we need to choose the most suitable tool based on the specific situation.
Do you have any questions about OOP? Or do you have any interesting experiences to share when using OOP? Feel free to leave a comment, and let’s discuss and learn together!
Finally, remember that practice is the most important in programming. So, get your hands dirty with code! Try refactoring your old projects using OOP or start a new one. Only through practice can we truly grasp the essence of OOP.
Have fun exploring the world of Python OOP! See you next time!
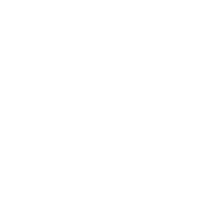