Dear Python programming enthusiasts, hello! Today we continue to explore the essence of Python object-oriented programming.
Let's quickly review the basics of object-oriented programming we've discussed before.
Classes and Objects
In Python, a Class is a blueprint or template for creating objects. We define a class that contains data attributes and behavior methods. Based on this class, we can create any number of specific object instances.
A class is an abstract concept, while an object is a concrete instance. For example, we can define a Player
class to represent characters in a game, including attributes like name
, health
, etc., and methods like move()
, attack()
, etc. Then we can create multiple Player
objects to represent different game characters.
Attributes and Methods
Objects contain attributes and methods. Attributes are the object's data, while methods define the object's behavior.
For example, a Player
object might have name
and health
attributes, as well as move()
and attack()
methods. We can access and modify object attributes and call methods using the .
operator.
Inheritance
Inheritance allows us to create a new class based on an existing class, enabling the new class to automatically possess the attributes and methods of the parent class. This greatly improves code reusability.
For instance, we can define an Enemy
class that inherits from the Player
class, so the Enemy
class automatically has the health
attribute and move()
method. We only need to add attributes and methods specific to the Enemy
class.
Encapsulation
Encapsulation is a core principle of object-oriented programming. It requires encapsulating an object's state and behavior together and restricting direct access to the object's internal data.
In Python, we typically use naming conventions to implement encapsulation. Attributes starting with a single underscore _
are considered protected, while those starting with double underscores __
are considered private.
Polymorphism
Polymorphism refers to the ability of subclasses to override methods of their parent class, thus implementing different behaviors. This diversity of behavior is polymorphism.
Polymorphism is another important feature of object-oriented programming, increasing code flexibility and extensibility. We can write code for the parent class rather than specific subclasses, achieving a unified programming approach.
That's a review of the basics of object-oriented programming. Now let's look at some more advanced concepts and design patterns.
Method Overriding
In inheritance relationships, subclasses can override methods of the parent class. This process of redefining methods to implement new behavior is called Method Overriding.
Method overriding is an important means of implementing polymorphism. Through overriding, we can execute different operation logic for different subclass objects.
For example, we have a Character
base class that defines a move()
method. Then we can override this method in the Player
and Enemy
subclasses to make their movement behaviors different.
class Character:
def move(self):
print("Character moves")
class Player(Character):
def move(self):
print("Player moves differently")
class Enemy(Character):
def move(self):
print("Enemy also moves in its own way")
player = Player()
player.move() # Output: Player moves differently
enemy = Enemy()
enemy.move() # Output: Enemy also moves in its own way
When overriding methods, the method signature (method name and parameters) in the subclass must be exactly the same as in the parent class. Otherwise, Python will treat it as a completely new method rather than an override.
Abstract Classes
An Abstract Class is a class that cannot be instantiated and is only used for inheritance. Abstract classes can contain abstract methods, which are methods that have declarations but no concrete implementations.
Subclasses inheriting from abstract classes must override all abstract methods, otherwise, the subclass will also become an abstract class.
In Python, we can define abstract classes and abstract methods using the abc
module. Here's an example:
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
@abstractmethod
def perimeter(self):
pass
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
def perimeter(self):
return 2 * (self.width + self.height)
rect = Rectangle(3, 4)
print(rect.area()) # Output: 12
print(rect.perimeter()) # Output: 14
Abstract classes provide a consistent interface for subclass implementations, enhancing code extensibility and maintainability.
Interfaces
An Interface is similar to a pure abstract class, but all methods in an interface must be abstract. Interfaces only define a set of method signatures, with the specific implementation provided by classes that implement the interface.
A class can implement multiple interfaces, thus acquiring all the abstract methods defined by multiple interfaces.
Although Python doesn't have specific interface syntax, we can use abstract base classes to simulate interfaces. Here's an example:
from abc import ABCMeta, abstractmethod
class Interface(metaclass=ABCMeta):
@abstractmethod
def method1(self):
pass
@abstractmethod
def method2(self):
pass
class ImplementingClass(Interface):
def method1(self):
print("Implementing method1")
def method2(self):
print("Implementing method2")
obj = ImplementingClass()
obj.method1() # Output: Implementing method1
obj.method2() # Output: Implementing method2
In the above example, Interface
is an abstract base class that defines two abstract methods. ImplementingClass
becomes a concrete class by inheriting from Interface
and implementing all abstract methods.
Interfaces provide clear contracts for code, enhancing program extensibility and maintainability.
Design patterns are typical solutions to common problems in software design. By using design patterns, we can improve code reusability, flexibility, and maintainability. Let's look at a common design pattern - the Singleton pattern.
Singleton Pattern
The Singleton Pattern ensures that a class has only one instance and provides a global access point to it. This is very useful when only one instance is needed to handle all work in a system.
In Python, we can implement the Singleton pattern through the module mechanism, because modules are created on first import and then cached for repeated use.
Here's a simple implementation of the Singleton pattern:
class Singleton(object):
_instance = None
def __new__(cls, *args, **kwargs):
if cls._instance is None:
cls._instance = super().__new__(cls)
return cls._instance
s1 = Singleton()
s2 = Singleton()
print(s1 is s2) # Output: True
In the above example, we override the __new__
method to ensure that the same instance is returned each time it's instantiated. The object is created on the first instantiation, and the already created instance is returned thereafter.
We can also use Python's module mechanism to implement the Singleton pattern:
class Singleton(object):
def foo(self):
pass
singleton = Singleton()
from singleton import singleton
obj = singleton
obj.foo()
The advantage of this approach is that the singleton object is automatically created when the module is loaded, and it's thread-safe.
Although the Singleton pattern is not commonly used in Python, it's still useful in certain scenarios, such as logging, configuration management, etc. It can help us save system resources and enhance object control capabilities.
Today we reviewed the basics of object-oriented programming and delved into advanced concepts such as polymorphism, abstract classes, interfaces, and design patterns.
With this knowledge, you can better design and implement Python programs that conform to software design principles. However, while theory is important, practice is key. Now, start writing some interesting object-oriented programs and put these concepts into practice!
The road of programming is long and arduous, let's move forward together and continue to grow in this exploration process. Happy coding, and welcome to continue following my blog. See you next time!
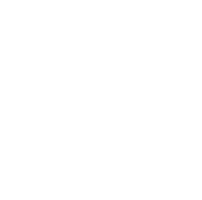