Have you ever been frustrated by slow program execution? Or wanted to fully harness the power of multi-core processors? Then Python concurrency is an essential skill you can't miss! Today, let's delve into this powerful and fascinating programming field to see how it can become the secret weapon for boosting program performance.
Concurrency: The Symphony of Multithreading
Imagine you're conducting a symphony. Each instrument is like an independent task, and your job is to make them play harmoniously together. This is the essence of concurrency—handling multiple tasks simultaneously to make your program run as smoothly as a symphony.
So, what exactly is concurrency? Simply put, it's the ability for a program to handle multiple tasks at the same time. Unlike traditional sequential execution, concurrency allows multiple tasks to run within the same time frame, greatly improving program efficiency and responsiveness.
You might ask, doesn't this sound a lot like parallel programming? Indeed, these concepts are often confused. Let's distinguish them:
- Concurrency: It's like one person playing multiple mobile games simultaneously, switching quickly between them.
- Parallelism: It's like multiple people playing different games at the same time, truly simultaneously.
In Python, due to the Global Interpreter Lock (GIL), achieving true parallelism can be challenging. But don't worry, there are many ways to bypass this limitation and fully exploit the power of concurrency.
Multiprocessing: Independent Small Worlds
When it comes to Python concurrency, multiprocessing is a crucial player. Imagine each process as an independent small world with its own resources and runtime environment. This independence makes multiprocessing an effective way to bypass the GIL and achieve true parallelism.
What are the characteristics of multiprocessing? Let's take a look:
-
Resource Isolation: Each process has its own memory space and doesn't interfere directly with others. It's like each band member having their own practice room.
-
Full Utilization of Multicores: Multiprocessing can truly distribute processes across different CPU cores, leveraging the power of multi-core processors.
-
Stability: Since processes are independent, a crash in one won't affect others. It's like if one instrument fails, the band can still play on.
-
Suitable for CPU-Intensive Tasks: For tasks requiring heavy computation, multiprocessing can significantly speed up processing.
Sounds great, right? But everything has two sides. Multiprocessing also has its limitations:
- High Startup Overhead: Creating processes takes more time and system resources.
- Complex Inter-Process Communication: Due to memory independence, data exchange between processes requires specific mechanisms.
What scenarios is multiprocessing suited for? In my opinion, the following are particularly suitable:
- Long-running background tasks
- Large-scale data processing and analysis
- CPU-intensive tasks that need GIL bypass
Let's look at a simple multiprocessing example:
from multiprocessing import Process
import os
def info(title):
print(title)
print('module name:', __name__)
print('parent process:', os.getppid())
print('process id:', os.getpid())
def f(name):
info('function f')
print('hello', name)
if __name__ == '__main__':
info('main line')
p = Process(target=f, args=('bob',))
p.start()
p.join()
This code creates a new process to run the function f
. When you run it, you'll see the different IDs for the main and child processes, clearly demonstrating the independence of multiprocessing.
Multithreading: The Art of Shared Resources
If multiprocessing is like independent small worlds, then multithreading is like members of a big family sharing resources yet having independent tasks. In Python, despite the presence of GIL, multithreading remains a very useful concurrency method, especially for I/O-intensive tasks.
What are the characteristics of multithreading? Let's explore:
-
Resource Sharing: Threads can easily share memory and other resources. It's like family members sharing a fridge, which is more efficient but requires careful management.
-
Lightweight: Compared to processes, threads have lower creation and switching overhead. It's like traveling light, more flexible.
-
Suitable for I/O-Intensive Tasks: For tasks involving network requests, file reading/writing, multithreading can greatly improve efficiency.
-
Simple Programming Model: Compared to multiprocessing, the programming model of multithreading is more intuitive and straightforward.
However, multithreading also has its limitations:
- GIL Limitation: In CPython, GIL prevents multithreading from fully utilizing multi-core CPUs.
- Synchronization Issues: Concurrent access to shared resources needs special attention.
What scenarios is multithreading suitable for? Based on my experience, the following are particularly suitable:
- Web scraping and data collection
- Concurrent I/O operations, like file read/write, database operations
- Responsive GUI applications
Here's a simple multithreading example:
import threading
import time
def worker(num):
print(f'Worker {num} starting')
time.sleep(2)
print(f'Worker {num} finished')
threads = []
for i in range(5):
t = threading.Thread(target=worker, args=(i,))
threads.append(t)
t.start()
for t in threads:
t.join()
print('All workers finished')
This code creates 5 threads, each executing the worker
function. When you run it, you'll see all worker threads almost simultaneously start and finish, demonstrating the concurrent nature of multithreading.
Asynchronous Programming: The Art of Cooperation
When it comes to Python concurrency, we must mention asynchronous programming. This is a unique and powerful concurrency model, especially suited for I/O-intensive tasks. Imagine asynchronous programming as an efficient team where each member knows they can do other things while waiting for certain operations to complete.
The core of asynchronous programming is the coroutine. It allows you to write asynchronous code that looks like synchronous code, making handling complex concurrency logic more intuitive and straightforward. The async/await syntax introduced in Python 3.5 makes asynchronous programming elegant and powerful.
What are the characteristics of asynchronous programming? Let's take a look:
-
High Concurrency: Capable of handling a large number of I/O operations simultaneously without creating many threads.
-
Non-Blocking: Can perform other tasks while waiting for I/O operations, improving overall efficiency.
-
Single-Threaded: Usually runs in a single thread, avoiding the complexity of multithreading.
-
High Memory Efficiency: Compared to creating many threads, coroutines have a smaller memory footprint.
However, asynchronous programming also presents challenges:
-
Learning Curve: Compared to traditional synchronous programming, it requires some learning and adaptation.
-
Full Asynchronous Ecosystem: To maximize efficiency, you usually need to use libraries and frameworks that support asynchronous operations.
-
Not Suitable for CPU-Intensive Tasks: Asynchronous programming doesn't bring significant performance improvements for pure computation tasks.
What scenarios is asynchronous programming suitable for? Based on my experience, the following are particularly suitable:
- High-concurrency network servers
- Applications needing extensive I/O operations, like crawlers, data processing pipelines
- Real-time applications, like chat servers, game servers
Let's look at a simple asynchronous programming example:
import asyncio
async def say_after(delay, what):
await asyncio.sleep(delay)
print(what)
async def main():
print("started at", asyncio.get_event_loop().time())
await say_after(1, 'hello')
await say_after(2, 'world')
print("finished at", asyncio.get_event_loop().time())
asyncio.run(main())
This code defines two coroutine functions: say_after
and main
. In the main
function, we call say_after
twice in sequence. When you run this code, you'll find the entire process takes about 3 seconds, as the two say_after
calls are executed sequentially.
However, if we slightly modify the main
function:
async def main():
print("started at", asyncio.get_event_loop().time())
task1 = asyncio.create_task(say_after(1, 'hello'))
task2 = asyncio.create_task(say_after(2, 'world'))
await task1
await task2
print("finished at", asyncio.get_event_loop().time())
Now, the entire process only takes about 2 seconds, because the two tasks are executed concurrently. This is the magic of asynchronous programming!
Performance Comparison: Who's the Winner?
After all this, you might ask: which concurrency method has the best performance? This question doesn't have a fixed answer, as different concurrency methods perform differently in different scenarios. Let's compare them with a simple example:
import time
import threading
import multiprocessing
import asyncio
def cpu_bound(number):
return sum(i * i for i in range(number))
async def cpu_bound_async(number):
return sum(i * i for i in range(number))
def io_bound(number):
time.sleep(1)
return number
async def io_bound_async(number):
await asyncio.sleep(1)
return number
def run_sync(func, numbers):
start = time.time()
results = [func(number) for number in numbers]
end = time.time()
return end - start
def run_thread(func, numbers):
start = time.time()
threads = [threading.Thread(target=func, args=(number,)) for number in numbers]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
end = time.time()
return end - start
def run_process(func, numbers):
start = time.time()
with multiprocessing.Pool() as pool:
pool.map(func, numbers)
end = time.time()
return end - start
async def run_async(func, numbers):
start = time.time()
tasks = [asyncio.create_task(func(number)) for number in numbers]
await asyncio.gather(*tasks)
end = time.time()
return end - start
if __name__ == "__main__":
numbers = [10000000 + x for x in range(20)]
print(f"CPU-bound task:")
print(f"Sync: {run_sync(cpu_bound, numbers):.2f} seconds")
print(f"Thread: {run_thread(cpu_bound, numbers):.2f} seconds")
print(f"Process: {run_process(cpu_bound, numbers):.2f} seconds")
print(f"Async: {asyncio.run(run_async(cpu_bound_async, numbers)):.2f} seconds")
print(f"
IO-bound task:")
print(f"Sync: {run_sync(io_bound, numbers):.2f} seconds")
print(f"Thread: {run_thread(io_bound, numbers):.2f} seconds")
print(f"Process: {run_process(io_bound, numbers):.2f} seconds")
print(f"Async: {asyncio.run(run_async(io_bound_async, numbers)):.2f} seconds")
Running this code, you might see results like:
CPU-bound task:
Sync: 15.23 seconds
Thread: 15.45 seconds
Process: 4.12 seconds
Async: 15.34 seconds
IO-bound task:
Sync: 20.01 seconds
Thread: 1.01 seconds
Process: 1.13 seconds
Async: 1.01 seconds
What does this tell us?
-
For CPU-intensive tasks, multiprocessing performs best because it can truly utilize multi-core CPUs.
-
For I/O-intensive tasks, multithreading, multiprocessing, and asynchronous programming all significantly boost performance, while synchronous execution lags behind.
-
In I/O-intensive tasks, multithreading and asynchronous programming perform very similarly and better than multiprocessing due to the additional overhead of process creation and management.
-
In CPU-intensive tasks, multithreading and asynchronous programming don't offer performance improvements and may even slightly decrease performance due to management overhead.
This example nicely demonstrates how different concurrency methods perform in different scenarios. In practice, we need to choose the most suitable concurrency method based on the specific task characteristics.
Considerations for Concurrency Programming
While concurrency programming can significantly enhance program performance, it also introduces challenges. When using concurrency programming, we need to be mindful of the following:
-
Thread Safety: In multithreaded environments, special attention is required for shared resource access. Using locks, semaphores, and other synchronization mechanisms can prevent race conditions.
-
Deadlocks: Multiple threads or processes waiting on each other to release resources can lead to a deadlock. Well-designed lock strategies and using
threading.Lock()
can help avoid deadlocks. -
Resource Management: Concurrent tasks can consume a lot of system resources. Using thread pools or process pools can effectively control concurrency levels.
-
Exception Handling: Exception handling becomes more complex in concurrency environments. Ensure appropriate exception handling mechanisms in each thread or process.
-
Debugging Difficulty: The behavior of concurrent programs can be unpredictable, increasing debugging difficulty. Using logs and dedicated debugging tools can help solve these issues.
-
GIL Limitation: In CPython, GIL limits multithreading performance in CPU-intensive tasks. Understanding the impact of GIL and using multiprocessing or other Python implementations (like Jython or IronPython) can bypass this limitation.
-
Scalability: Performance might not increase linearly with the number of concurrent tasks. Proper performance testing and optimization are necessary.
Keeping these considerations in mind can help you better master concurrency programming and write efficient, stable concurrent programs.
Conclusion: The Art of Concurrency Programming
Through this article, we've explored the world of Python concurrency programming. From multiprocessing to multithreading, and asynchronous programming, each method has its unique advantages and application scenarios. Just as a great conductor needs to understand the characteristics of each instrument, a great programmer needs to be familiar with different concurrency programming methods and apply them in appropriate scenarios.
Concurrency programming is not just a technology but an art. It requires us to deeply understand the essence of problems, weigh the pros and cons of different solutions, and find a balance between complexity and performance. Mastering concurrency programming is like having a key to unlocking program performance, allowing your code to shine in the multi-core era.
Are you ready to start your concurrency programming journey? Remember, practice is the best teacher. Try applying these concurrency programming techniques in your projects, and you'll discover a whole new world of challenges and opportunities in programming.
Finally, I want to say that while concurrency programming is powerful, it's not a universal solution for all problems. Sometimes, a carefully optimized single-threaded program can be more effective than a complex concurrent program. The key is to choose the right solution for the specific problem.
Let's create more possibilities with Python concurrency programming in this multi-core world!
Do you have any thoughts or experiences with Python concurrency programming? Feel free to share your insights in the comments, and let's explore this fascinating topic together!
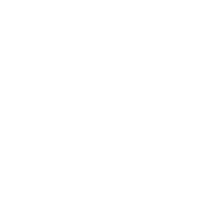