Hello everyone, welcome to my Python programming blog! Today we're going to talk about concurrent programming in Python. I believe many people have some doubts and confusion about concurrent programming, but don't worry, I'll try to explain it clearly in simple terms. Let's get started!
What is Concurrency?
First, we need to understand what concurrent programming is. Do you know why computers are so fast? It's because the CPU can execute multiple tasks simultaneously, and this technique of executing multiple tasks at the same time is called concurrency.
However, you might say: "My computer only has one CPU, how can it execute multiple tasks simultaneously?" Don't rush, let me explain.
Imagine you're eating hot pot, eating meat and cooking vegetables at the same time. Your mouth is eating meat, your hand is cooking vegetables, and the two actions are happening simultaneously. This is an example of concurrency! The CPU works the same way, it can switch to another task while executing one task, then switch back to the first task, quickly switching between multiple tasks, making it look like it's executing multiple tasks simultaneously.
Multithreading and Multiprocessing
So how do we implement concurrent programming in Python? There are mainly two ways: multithreading and multiprocessing.
Multithreading is starting multiple threads within one process, and these threads share the same memory space. This method is suitable for handling some IO-intensive tasks, such as network requests, file reading and writing, etc. However, due to the existence of the Global Interpreter Lock (GIL) in Python, only one thread can run at a time, so multithreading is not suitable for CPU-intensive tasks.
Multiprocessing is starting multiple processes, each process having its own independent memory space. This method is suitable for handling CPU-intensive tasks and can fully utilize the advantages of multi-core CPUs. However, inter-process communication is relatively complex, and the overhead is also larger than threads.
So, if your task is IO-intensive, such as web crawling, sending network requests, etc., then use multithreading. If it's a CPU-intensive task, such as scientific computing, image processing, etc., then using multiprocessing is more appropriate.
Concurrent Programming in Practice
Alright, we've talked about so much theory, now let's put it into practice! Python provides the concurrent.futures
module in the standard library, which can easily implement concurrent programming.
ThreadPoolExecutor
First, let's look at an example using ThreadPoolExecutor
:
from concurrent.futures import ThreadPoolExecutor
def get_something():
# Simulate time-consuming operation
import time
time.sleep(2)
return "I am the result"
with ThreadPoolExecutor() as executor:
future1 = executor.submit(get_something)
future2 = executor.submit(get_something)
result1 = future1.result()
result2 = future2.result()
print(result1, result2)
In this example, we create a ThreadPoolExecutor
object, then use the submit
method to submit two tasks. The submit
method will immediately return a Future
object, and we can get the result of the task through the result
method.
You might ask: "Why use the with
statement?" This is because ThreadPoolExecutor
will occupy some system resources, and using the with
statement can ensure that these resources are automatically released after execution.
When you run this program, you'll find that the two tasks are executed in parallel, so the total execution time is shorter than the execution time of a single task.
ProcessPoolExecutor
If you want to take advantage of multi-core CPUs, you can use ProcessPoolExecutor
. The usage is similar to ThreadPoolExecutor
, you just need to change the imported module to ProcessPoolExecutor
. However, note that due to the overhead of inter-process communication, using multiprocessing may be slower than single-process for some simple tasks.
Asynchronous Programming
In addition to using thread pools and process pools, we can also use Python's asyncio
module to implement asynchronous programming. The working principle of asyncio
is similar to multithreading, but it is implemented through an event loop, not operating system-level thread switching. This makes the performance of asyncio
superior to traditional multithreading models.
Here's an example using asyncio
:
import asyncio
async def get_something():
# Simulate time-consuming operation
await asyncio.sleep(2)
return "I am the result"
async def main():
task1 = asyncio.create_task(get_something())
task2 = asyncio.create_task(get_something())
result1 = await task1
result2 = await task2
print(result1, result2)
asyncio.run(main())
In this example, we define a get_something
coroutine function that uses asyncio.sleep
to simulate a time-consuming operation. In the main
function, we create two tasks and use the await
keyword to wait for them to complete. Finally, we use the asyncio.run
function to start the event loop.
You might ask: "Why use async/await
?" This is because Python's coroutines are implemented through yield, and using async/await
can make the code look more concise and intuitive.
Summary
Alright, that's all for today's sharing! We learned what concurrent programming is, and the two main ways to implement concurrent programming in Python: multithreading and multiprocessing. We also practiced how to implement concurrent programming using the concurrent.futures
module and the asyncio
module.
Concurrent programming is a very important concept that can help us improve the performance and responsiveness of programs. However, concurrent programming also has some pitfalls and challenges, such as deadlocks, race conditions, etc. So in actual development, we need to be extra careful.
If you have any questions about concurrent programming, feel free to leave a comment for discussion! You're also welcome to share your own experiences and insights. The road of programming is long and arduous, let's move forward together and grow together!
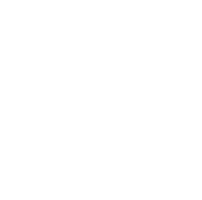