Hey, dear Python enthusiasts! Today, we're going to discuss a fascinating topic—the application of Python programming in data science. Do you often hear the term "data science" but aren't quite sure what it means? Don't worry, let's unravel its mysteries and see how Python shines in this field.
Fundamentals
First, we need to master some basics. Did you know? Learning programming is like learning a new language. We start with basic "vocabulary" and "grammar," then slowly build complex "sentences" and "paragraphs."
Playing with Loops
Loops might sound a bit dull, but they are a powerful tool in programming! Imagine if you need to repeat something 100 times. Would you do it manually? That would be exhausting! This is where loops come in handy.
for i in range(100):
print(f"This is repetition number {i+1}")
See, with just a few lines of code, we can easily complete 100 repetitions. Isn't that amazing?
Conditional Statements
In life, we often need to make choices. Programming is no different! Conditional statements are how we make decisions in our code.
temperature = 25
if temperature > 30:
print("It's really hot today!")
elif temperature < 10:
print("It's a bit cold today.")
else:
print("The weather is nice today!")
With these conditional checks, our program can respond differently to various situations. Doesn't it seem like the program has become a bit "smarter"?
Unveiling Data Structures
In data science, we often need to handle large amounts of data. Choosing the right data structure becomes crucial. Python provides us with various powerful data structures; let's explore them!
The Magic of Lists
Lists are one of the most commonly used data structures in Python. They are like a treasure chest that can hold various items.
my_list = [1, 2, 3, "Python", [4, 5, 6]]
print(my_list[3]) # Output: Python
See, we can store numbers, strings, and even another list in a list! This flexibility makes lists very convenient for handling various data types.
The Charm of Dictionaries
If lists are treasure chests, dictionaries are like an encyclopedia full of entries, each with a unique "key" and corresponding "value."
my_dict = {"name": "Alice", "age": 25, "skills": ["Python", "Data Analysis"]}
print(my_dict["skills"]) # Output: ['Python', 'Data Analysis']
With dictionaries, we can organize and access data more intuitively. They are often useful for handling complex data structures.
The Uniqueness of Sets
A set, as the name suggests, is a collection of unique elements. Its features are that elements are not repeated and are unordered.
my_set = {1, 2, 3, 3, 4, 4, 5}
print(my_set) # Output: {1, 2, 3, 4, 5}
See, duplicate elements are automatically removed. When you need to deduplicate or quickly check for the existence of an element, sets are a good choice.
The Stability of Tuples
Finally, let's talk about tuples. Tuples are similar to lists but with one key difference: once created, their contents cannot be changed.
my_tuple = (1, 2, 3)
This "once set, cannot be changed" feature makes tuples particularly useful in scenarios where you need to ensure data isn't accidentally modified.
Functions and Unit Testing
By now, you might be thinking, isn't it tedious to write so much code each time? Don't worry, functions come to the rescue!
The Magic of Functions
Functions are like small "programs" that let us package frequently used code into reusable units.
def greet(name):
return f"Hello, {name}! Welcome to the world of Python."
print(greet("Alice")) # Output: Hello, Alice! Welcome to the world of Python.
By defining functions, we can greatly improve the reusability and readability of our code. Doesn't it feel like the code suddenly became more organized?
Unit Testing for Assurance
After writing functions, you might wonder, how do I know if my function is correct? That's when unit testing comes into play.
import unittest
class TestGreet(unittest.TestCase):
def test_greet(self):
self.assertEqual(greet("Bob"), "Hello, Bob! Welcome to the world of Python.")
if __name__ == '__main__':
unittest.main()
With unit testing, we can automatically verify that a function's output matches our expectations. This way, we can be more confident that our code is correct.
NumPy: A Reliable Assistant in Data Science
Speaking of data science, we must mention the powerful NumPy library. NumPy provides efficient array operations and mathematical functions, making data processing a breeze.
Unveiling Array Operations
The core of NumPy is the ndarray object, which lets us handle multi-dimensional arrays easily.
import numpy as np
arr = np.array([[1, 2, 3], [4, 5, 6]])
print(arr)
print(arr.shape) # Output: (2, 3)
reshaped_arr = arr.reshape(3, 2)
print(reshaped_arr)
See, we can easily create, view, and reshape arrays. This is very useful when dealing with large-scale data.
The Magic of Mathematical Calculations
NumPy not only handles arrays but also provides numerous mathematical functions, simplifying complex calculations.
arr = np.array([1, 2, 3, 4, 5])
print(np.mean(arr)) # Output: 3.0
print(np.std(arr)) # Output: 1.4142135623730951
print(np.max(arr), np.min(arr)) # Output: 5 1
matrix1 = np.array([[1, 2], [3, 4]])
matrix2 = np.array([[5, 6], [7, 8]])
print(np.dot(matrix1, matrix2))
See, with just a few lines of code, we completed the calculation of the average, standard deviation, and even complex matrix multiplication. NumPy truly makes mathematical calculations so simple!
Pandas: The Swiss Army Knife of Data Processing
If NumPy is the foundation of data science, then Pandas is the "Swiss Army Knife" built on that foundation. It provides powerful data structures and data analysis tools, allowing us to easily handle various types of data.
Series and DataFrame: Data's Best Friends
Pandas' two main data structures are Series and DataFrame. A Series is like a one-dimensional array with labels, while a DataFrame can be seen as a two-dimensional table composed of multiple Series.
import pandas as pd
s = pd.Series([1, 3, 5, np.nan, 6, 8])
print(s)
df = pd.DataFrame({
'A': [1, 2, 3, 4],
'B': pd.date_range('20210101', periods=4),
'C': pd.Series(1, index=list(range(4)), dtype='float32'),
'D': np.array([3] * 4, dtype='int32'),
'E': pd.Categorical(["test", "train", "test", "train"]),
'F': 'foo'
})
print(df)
See, we can easily create DataFrames containing different types of data. This flexibility allows us to handle complex real-world datasets.
The Magic of Data Processing and Analysis
Pandas not only provides powerful data structures but also rich data processing and analysis functions.
df = pd.read_csv('data.csv')
print(df.info())
print(df.describe())
print(df['column_name'])
print(df[df['column_name'] > 5])
print(df.groupby('category')['value'].mean())
df.fillna(0, inplace=True)
df.sort_values('column_name', ascending=False, inplace=True)
This is just the tip of the iceberg of Pandas' capabilities. With these operations, we can quickly understand the data structure, clean the data, and perform statistical analysis. Doesn't it make data processing feel so simple?
Code Quality: Taking Your Code to the Next Level
It's easy to write code that runs, but writing high-quality code requires some skills. Let's see how to improve code quality, making your code more elegant and efficient.
The Art of Code Abstraction
Code abstraction is a technique that encapsulates complex logic into simple interfaces. Through the abstraction of functions and classes, we can make code more modular and easier to understand and maintain.
Function Abstraction
Function abstraction is the most basic form of code abstraction. Let's look at an example:
def process_data(data):
result = []
for item in data:
if item > 0:
result.append(item * 2)
else:
result.append(item * -1)
return result
def double_positive(x):
return x * 2 if x > 0 else x
def negate_negative(x):
return x * -1 if x < 0 else x
def process_data(data):
return [double_positive(negate_negative(x)) for x in data]
See the difference? By splitting the logic into small functions, our code becomes clearer, with each function having a clear responsibility.
Class Abstraction
Class abstraction goes a step further, encapsulating both behavior (methods) and state (attributes).
class DataProcessor:
def __init__(self, data):
self.data = data
def double_positive(self, x):
return x * 2 if x > 0 else x
def negate_negative(self, x):
return x * -1 if x < 0 else x
def process(self):
return [self.double_positive(self.negate_negative(x)) for x in self.data]
processor = DataProcessor([1, -2, 3, -4, 5])
result = processor.process()
print(result) # Output: [2, 2, 6, 4, 10]
Through class abstraction, we combine data and methods for processing data into a complete "object." This approach is especially suitable for handling complex data structures and algorithms.
Code Readability: Making Your Code Speak
The readability of code directly affects how easily others (including future you) can understand and maintain it. Here are some tips to enhance code readability:
- Use meaningful variable names: ```python # Poor practice x = 5 y = 10 z = x + y
# Better practice apples = 5 oranges = 10 total_fruits = apples + oranges ```
-
Add appropriate comments:
python # Calculate the total number of fruits total_fruits = apples + oranges
-
Use blank lines to separate logical blocks: ```python def complex_function(): # Initialize variables x = 0 y = 0
# Perform some complex calculations for i in range(10): x += i y += i ** 2
# Return results return x, y ```
-
Follow PEP 8 coding standards: PEP 8 is Python's official style guide; following it can make your code more Pythonic.
Code Reusability: Don't Reinvent the Wheel
Code reuse is key to improving development efficiency. By creating reusable functions and classes, we can avoid rewriting similar code.
- Create generic functions: ```python def calculate_average(numbers): return sum(numbers) / len(numbers)
# Can be used in multiple places avg_scores = calculate_average([85, 90, 78, 92]) avg_temperatures = calculate_average([23.5, 24.1, 22.8, 25.0]) ```
- Use decorators: Decorators are a powerful way to reuse code, adding new functionality without modifying the original function. ```python def log_function_call(func): def wrapper(args, kwargs): print(f"Calling function: {func.name}") return func(args, **kwargs) return wrapper
@log_function_call def add(a, b): return a + b
result = add(3, 5) # Prints "Calling function: add" ```
-
Create reusable classes: ```python class DataValidator: @staticmethod def is_positive(value): return value > 0
@staticmethod def is_in_range(value, min_value, max_value): return min_value <= value <= max_value
# This class can be used in multiple places validator = DataValidator() if validator.is_positive(user_input) and validator.is_in_range(user_input, 1, 100): print("Input is valid") ```
By applying these techniques, we can significantly improve code quality and efficiency. Remember, writing code is not just for computers to understand but for people to understand as well. A good programmer not only solves problems but also writes code that is easy to understand and maintain.
Conclusion
Wow, we've learned a lot today! From Python basics to powerful data processing tools and techniques for improving code quality, we now have a comprehensive understanding of Python's application in data science.
Have you noticed that Python is like a Swiss Army Knife, helping us easily tackle various data processing and analysis tasks? Whether it's simple mathematical calculations or complex data processing, Python handles it all with ease.
However, to truly master these skills, just reading isn't enough. You need to practice and try solving real-world problems with the knowledge gained today. For example, you can try analyzing data about your favorite musician's songs to see if you can find any interesting patterns. Or, you could use Python to analyze your daily expenses and understand your spending habits.
Remember, programming is like learning a new language; it requires constant practice and application. Don't be afraid to make mistakes; each mistake is a learning opportunity. Take it slow, believe in yourself, and you will become an outstanding Python data scientist!
So, are you ready to start your Python data science journey? Let's explore this world of endless possibilities together!
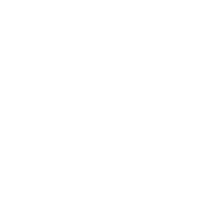
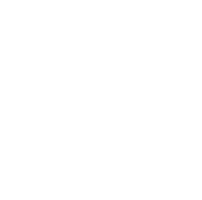