Hello, Python enthusiasts! Today, we're embarking on a fascinating journey, from the basics of loop statements to the exciting world of data science. Ready? Let's get started!
The Dance of Loops
Remember how you felt when you first encountered loops? A bit dizzy? Don't worry, let's talk about this interesting topic.
Loops in Python are like a magic box, allowing you to repeat tasks. Imagine you have a bunch of apples to wash. How would you do it? Of course, wash them one by one, right? That's the essence of loops!
Here's a simple example:
fruits = ["apple", "banana", "orange", "pear"]
for fruit in fruits:
print(f"I'm washing {fruit}")
See that? We use a for
loop to "wash" each fruit. This code will output:
I'm washing apple
I'm washing banana
I'm washing orange
I'm washing pear
Isn't it magical? Imagine having 100 types of fruits; the loop handles it easily without writing 100 lines of code!
But wait, there are more tricks with loops. For instance, what if we only want to wash even-numbered fruits? That's where the if
statement comes in:
for i in range(len(fruits)):
if i % 2 == 0:
print(f"I'm washing the {i+1}th fruit: {fruits[i]}")
This code will output:
I'm washing the 1st fruit: apple
I'm washing the 3rd fruit: orange
See? We use if i % 2 == 0
to check for even positions. That's the charm of conditional statements!
The Ocean of Data
After loops, let's talk about data structures. In Python, data structures are like different types of containers for storing data.
Lists: The Versatile Treasure Box
Lists are perhaps the most commonly used data structure in Python. They're like a treasure box where you can put anything and modify it anytime.
my_list = [1, "hello", 3.14, [1, 2, 3]]
print(my_list[1]) # Output: hello
my_list.append("Python")
print(my_list) # Output: [1, "hello", 3.14, [1, 2, 3], "Python"]
See, we can even place another list inside a list! This flexibility makes lists a handy tool for Python programmers.
Dictionaries: The Smart Label Maker
If lists are treasure boxes, dictionaries are like smart label makers. You can label items and quickly find them.
student = {
"name": "Xiao Ming",
"age": 18,
"scores": {"math": 95, "english": 88}
}
print(student["name"]) # Output: Xiao Ming
print(student["scores"]["math"]) # Output: 95
See? We can even nest dictionaries! This structure is perfect for representing complex data relationships.
Tuples: Strong as a Rock
Tuples are like sealed lists. Once created, you can't change their contents.
my_tuple = (1, 2, 3)
You might wonder why we need such a "stubborn" data structure. Imagine having data you don't want accidentally modified; tuples are the best choice!
Sets: Unique and Magical
Sets are like a magic bag that automatically removes duplicates.
my_set = {1, 2, 2, 3, 3, 3}
print(my_set) # Output: {1, 2, 3}
Sets are great for deduplication or membership tests. Just one line to check if an element is in the set:
print(2 in my_set) # Output: True
Isn't it cool?
Functions: Building Blocks of Code
After data structures, let's talk about functions. Functions are like building blocks of code, allowing you to package operations for reuse.
Here's a simple example:
def greet(name):
"""This function greets a person"""
return f"Hello, {name}!"
print(greet("Xiao Ming")) # Output: Hello, Xiao Ming!
See, we defined a greet
function that takes a name as a parameter and returns a greeting. We also added a docstring to describe the function's purpose. It's a good habit to help others (and future you) understand the function's role.
But how do we ensure our function works as expected? Enter unit tests.
def test_greet():
assert greet("Xiao Ming") == "Hello, Xiao Ming!"
assert greet("Xiao Hong") == "Hello, Xiao Hong!"
print("All tests passed!")
test_greet()
This test function checks if greet
handles different inputs correctly. If all assertions pass, our function works fine.
Abstraction: The Art of Code
Speaking of functions, we must mention abstraction. Abstraction simplifies complexity by focusing only on core parts. In programming, we often use functions and classes for abstraction.
Here's an example:
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
def perimeter(self):
return 2 * (self.width + self.height)
rect = Rectangle(5, 3)
print(f"Area: {rect.area()}") # Output: Area: 15
print(f"Perimeter: {rect.perimeter()}") # Output: Perimeter: 16
The Rectangle
class abstracts the concept of a rectangle. We can create different rectangle objects and calculate their area and perimeter without rewriting formulas. That's the power of abstraction!
Data Science: Python's Playground
After Python basics, let's discuss its application in data science. Python is a favorite in data science, thanks largely to powerful libraries like NumPy and Pandas.
NumPy: The Superhero of Numerical Computation
NumPy provides a powerful multi-dimensional array object and tools to manipulate these arrays. Here's an example:
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
mean = np.mean(arr)
print(f"Mean: {mean}") # Output: Mean: 3.0
std = np.std(arr)
print(f"Standard Deviation: {std}") # Output: Standard Deviation: 1.4142135623730951
See? Just a few lines to complete common statistical calculations. Pure Python would be much more complex.
Pandas: The Swiss Army Knife of Data Processing
If NumPy is the superhero of numerical computation, Pandas is the Swiss Army knife of data processing. It offers powerful data structures and analysis tools.
Here's an example:
import pandas as pd
df = pd.DataFrame({
'Name': ['Xiao Ming', 'Xiao Hong', 'Xiao Hua'],
'Age': [18, 17, 19],
'Score': [85, 92, 78]
})
average_score = df['Score'].mean()
print(f"Average Score: {average_score}") # Output: Average Score: 85.0
top_student = df.loc[df['Score'].idxmax()]
print(f"Top Student: {top_student['Name']}") # Output: Top Student: Xiao Hong
See? We easily created a DataFrame with student information and performed some data analysis. Pandas simplifies complex data processing.
The Magic of Data Processing
In real-world data science projects, we often handle various data types. Pandas offers powerful tools for numerical, string, and datetime data.
Numerical Data Processing
Suppose we have exam scores and want to standardize them (convert to z-scores):
import pandas as pd
import numpy as np
scores = pd.Series([85, 92, 78, 90, 88])
z_scores = (scores - scores.mean()) / scores.std()
print(z_scores)
This code outputs standardized scores. Standardization is common in data preprocessing, making data across scales comparable.
String Processing
Pandas also offers powerful string processing. For example, we can easily extract specific parts of a string:
names = pd.Series(['Zhang, Ming', 'Li, Hong', 'Wang, Hua'])
last_names = names.str.split(',').str[0]
print(last_names)
This code extracts last names. Such operations are common when handling text data.
Datetime Processing
Handling datetime data is also common in data science. Pandas makes it easy:
dates = pd.date_range(start='2023-01-01', end='2023-12-31', freq='D')
df = pd.DataFrame({'date': dates})
df['year'] = df['date'].dt.year
df['month'] = df['date'].dt.month
df['day'] = df['date'].dt.day
print(df.head())
This code creates a DataFrame with all 2023 dates and extracts year, month, and day information.
Best Practices in Programming
After so many technical details, let's talk about "soft" topics: best practices in programming. These might seem less important but are crucial for writing high-quality code.
Code Readability: Writing for Future You
Ever found code you wrote months ago incomprehensible? That's why code readability is vital.
Naming Conventions
Good names are like good labels, immediately conveying what the code does. For example:
def f(x, y):
return x + y
def add_numbers(first_number, second_number):
return first_number + second_number
See the difference? Good naming makes code self-explanatory, reducing the need for comments.
Comments and Documentation
While good naming reduces the need for comments, appropriate comments and documentation remain crucial. Especially for complex functions or classes, a good docstring greatly enhances readability:
def calculate_bmi(weight, height):
"""
Calculate Body Mass Index (BMI)
Parameters:
weight (float): Weight in kilograms
height (float): Height in meters
Returns:
float: BMI value
"""
return weight / (height ** 2)
This way, anyone (including you) can quickly understand the function's purpose and usage by checking the docstring.
Coding Style: Beautiful Code
Just as writing has formats, programming has its "formats." In Python, we usually follow the PEP 8 guidelines.
PEP 8 Guidelines
PEP 8 is Python's official style guide, containing many specific rules, such as:
- Use 4 spaces per indentation level
- Limit lines to 79 characters
- Use spaces around binary operators
Let's see an example:
def long_function_name(var_one,var_two,
var_three,var_four):
print(var_one)
def long_function_name(
var_one, var_two, var_three,
var_four):
print(var_one)
These small changes make code more consistent and readable.
Code Organization and Structure
Good code organization makes projects clearer. Generally, we group related functions and classes into modules. For example:
def clean_data(data):
# Code for data cleaning
def transform_data(data):
# Code for data transformation
from data_processing import clean_data, transform_data
def analyze_data(data):
cleaned_data = clean_data(data)
transformed_data = transform_data(cleaned_data)
# Code for data analysis
This organization makes the code more modular, maintainable, and extensible.
Conclusion: The Infinite Possibilities of Python
Our Python journey ends here. From basic loops and conditionals to data structures, functions, abstraction, and finally, the realm of data science, we've seen Python's power and flexibility.
But this is just the beginning. The world of Python is vast, with many unexplored areas. We haven't even touched on Python's applications in AI, machine learning, web development, and game development.
Have you thought about what else Python can do? Maybe you have some ideas? Feel free to share in the comments, and let's explore Python's infinite possibilities together!
Remember, programming is not just about writing code; it's the art of problem-solving. Stay curious, keep learning and practicing, and you'll discover more fun and opportunities in the world of Python.
So, are you ready to start your Python adventure? Let's explore this world full of possibilities together!
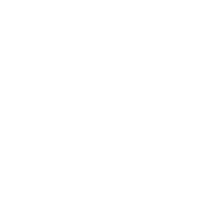
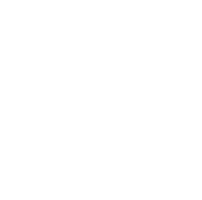