Hello, dear Python enthusiasts! Today, let's talk about a very interesting and powerful feature in Python—decorators. Have you ever wondered how to add new functionality to a function without modifying the original function? Or have you ever encountered needing to repeat the same operation on multiple functions? If so, decorators are the tool tailored for you!
What Are They
As the name suggests, decorators are tools used to "decorate" functions or classes. They allow us to add new functionality to functions or classes without modifying the original code. Doesn't that sound amazing? Let's unveil the mystery of decorators step by step!
First, we need to understand an important concept: in Python, functions are first-class citizens. This means functions can be assigned to variables, passed as arguments to other functions, and returned as values. This provides the foundation for using decorators.
Basic Usage
Let's start with a simple example. Suppose we have a function that calculates the sum of two numbers:
def add(a, b):
return a + b
result = add(3, 5)
print(result) # Output: 8
Now, if we want to print some log information every time this function is called, but don't want to modify the original function, what should we do? This is where decorators come into play:
def log_decorator(func):
def wrapper(*args, **kwargs):
print(f"Calling function: {func.__name__}")
result = func(*args, **kwargs)
print(f"Function {func.__name__} returned: {result}")
return result
return wrapper
@log_decorator
def add(a, b):
return a + b
result = add(3, 5)
print(result) # Output: 8
See that? We just added @log_decorator
above the original function, and we achieved the logging functionality without changing a single line of the original add
function's code! That's the magic of decorators.
How It Works
You might ask, how is this achieved? Let me explain.
When we use @log_decorator
to decorate the add
function, the Python interpreter actually does the following operation:
add = log_decorator(add)
This line of code means passing the original add
function as an argument to the log_decorator
function, and then assigning the new function returned by log_decorator
back to add
.
Inside the log_decorator
function, we define a new function wrapper
. This wrapper
function accepts any number of positional arguments (*args
) and keyword arguments (**kwargs
), so it can adapt to any function's parameters. In the wrapper
function, we first print the log, then call the original function, and finally print the return value and return the result.
This way, we successfully added new functionality to the function without modifying the original function. Isn't it clever?
Decorators with Arguments
The power of decorators doesn't stop there. We can also create decorators with arguments, which allows us to control the behavior of the decorator more flexibly. Let's see an example:
def repeat(times):
def decorator(func):
def wrapper(*args, **kwargs):
for _ in range(times):
result = func(*args, **kwargs)
return result
return wrapper
return decorator
@repeat(3)
def greet(name):
print(f"Hello, {name}!")
greet("Alice")
In this example, we create a repeat
decorator that can accept a parameter to control the number of times the function is called. We decorate the greet
function with @repeat(3)
, so the greet
function will be called three times consecutively.
You might have noticed that a decorator with arguments is actually a function that returns a decorator. Here, the repeat
function returns the actual decorator decorator
, and decorator
returns the wrapper
function. This nested structure allows us to control the behavior of the decorator very flexibly.
Classes as Decorators
Besides functions, we can also use classes to create decorators. This is especially useful when maintaining state is required. Let's see an example:
class CountCalls:
def __init__(self, func):
self.func = func
self.count = 0
def __call__(self, *args, **kwargs):
self.count += 1
print(f"{self.func.__name__} has been called {self.count} times")
return self.func(*args, **kwargs)
@CountCalls
def say_hello():
print("Hello!")
say_hello()
say_hello()
say_hello()
In this example, we create a CountCalls
class as a decorator. The __init__
method of this class receives the decorated function as a parameter, and the __call__
method implements the main logic of the decorator. Every time the decorated function is called, the __call__
method is invoked, allowing us to easily record the number of times the function has been called.
The main advantage of using classes as decorators is that we can maintain state more conveniently (in this example, the count of calls). This is very useful in some scenarios, such as caching, timers, etc.
Practical Applications
After discussing so much theory, you might ask: What are the uses of decorators in actual development? Let me give you a few examples:
-
Logging: Just like our initial example, decorators can be used to add logging functionality to functions, facilitating debugging and monitoring.
-
Performance Measurement: We can create a decorator to measure the execution time of a function.
import time
def timing_decorator(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"{func.__name__} took {end_time - start_time:.2f} seconds to execute")
return result
return wrapper
@timing_decorator
def slow_function():
time.sleep(2)
slow_function()
- Caching: Decorators can be used to implement a simple caching mechanism to avoid repeated calculations.
def memoize(func):
cache = {}
def wrapper(*args):
if args in cache:
return cache[args]
result = func(*args)
cache[args] = result
return result
return wrapper
@memoize
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
print(fibonacci(100)) # This calculation will be fast because intermediate results are cached
- Permission Checks: In web applications, we can use decorators to check whether a user has permission to perform an operation.
def admin_required(func):
def wrapper(user, *args, **kwargs):
if not user.is_admin:
raise PermissionError("Admin privileges required")
return func(user, *args, **kwargs)
return wrapper
@admin_required
def delete_user(admin, user_id):
# Code to delete user
pass
- Retry Mechanism: When a function fails to execute, we can use a decorator to automatically retry.
import time
def retry(max_attempts, delay=2):
def decorator(func):
def wrapper(*args, **kwargs):
attempts = 0
while attempts < max_attempts:
try:
return func(*args, **kwargs)
except Exception as e:
print(f"Attempt {attempts + 1} failed: {str(e)}")
attempts += 1
time.sleep(delay)
raise Exception(f"Function failed after {max_attempts} attempts")
return wrapper
return decorator
@retry(max_attempts=3, delay=2)
def unstable_function():
import random
if random.random() < 0.7:
raise Exception("Random error")
return "Success!"
print(unstable_function())
These are just the tip of the iceberg for decorator applications. In actual development, you'll find decorators can help you solve various problems, making your code more concise, elegant, and maintainable.
Considerations
While decorators are very powerful, there are some issues to be aware of when using them:
- Function Metadata: Using decorators might change the original function's metadata (such as the function name, docstring, etc.). We can use the
functools.wraps
decorator to preserve this information:
from functools import wraps
def my_decorator(func):
@wraps(func)
def wrapper(*args, **kwargs):
"""This is the wrapper function"""
return func(*args, **kwargs)
return wrapper
@my_decorator
def my_function():
"""This is the original function"""
pass
print(my_function.__name__) # Output: my_function
print(my_function.__doc__) # Output: This is the original function
- Execution Order: When using multiple decorators, note that their execution order is from bottom to top.
def decorator1(func):
print("decorator1")
return func
def decorator2(func):
print("decorator2")
return func
@decorator1
@decorator2
def my_function():
pass
my_function()
-
Performance Impact: Although decorators can make code more concise, overuse might affect performance. Each function call adds another layer of function calls, which could be problematic in high-performance scenarios.
-
Debugging Difficulty: Using decorators might make debugging more difficult, as the actual code being executed is wrapped in the decorator.
Deep Dive
If you've mastered the basic usage of decorators, let's explore some more advanced topics:
- Decorator Chains: We can apply multiple decorators to the same function, forming a decorator chain.
def bold(func):
def wrapper():
return "<b>" + func() + "</b>"
return wrapper
def italic(func):
def wrapper():
return "<i>" + func() + "</i>"
return wrapper
@bold
@italic
def greet():
return "Hello, world!"
print(greet()) # Output: <b><i>Hello, world!</i></b>
In this example, the greet
function is first decorated by the italic
decorator and then by the bold
decorator. The execution order is from inside out, so the final result is bold wrapping italic.
- Class Method Decorators: We can decorate not only regular functions but also class methods. Python provides built-in decorators like
@classmethod
and@staticmethod
to define special class methods.
class MyClass:
@classmethod
def class_method(cls):
print("This is a class method")
@staticmethod
def static_method():
print("This is a static method")
MyClass.class_method() # Output: This is a class method
MyClass.static_method() # Output: This is a static method
- Decorator Factory: We can create functions that return decorators, allowing us to customize the behavior of decorators more flexibly.
def prefix_decorator(prefix):
def decorator(func):
def wrapper(*args, **kwargs):
return prefix + str(func(*args, **kwargs))
return wrapper
return decorator
@prefix_decorator("Result: ")
def add(a, b):
return a + b
print(add(3, 5)) # Output: Result: 8
- Decorators with Optional Parameters: We can create decorators that can be used directly or accept parameters.
def repeat(func=None, times=2):
def decorator(f):
def wrapper(*args, **kwargs):
for _ in range(times):
result = f(*args, **kwargs)
return result
return wrapper
if func is None:
return decorator
else:
return decorator(func)
@repeat
def say_hello():
print("Hello!")
@repeat(times=3)
def say_hi():
print("Hi!")
say_hello() # Outputs "Hello!" twice
say_hi() # Outputs "Hi!" three times
In this example, the repeat
decorator can be used directly (@repeat
) or accept parameters (@repeat(times=3)
).
- Preserving Function Signature: In some cases, we might need to preserve the signature of the decorated function. Python's
inspect
module provides some useful tools:
import inspect
from functools import wraps
def preserve_signature(func):
@wraps(func)
def wrapper(*args, **kwargs):
return func(*args, **kwargs)
# Copy the original function's parameter signature
wrapper.__signature__ = inspect.signature(func)
return wrapper
@preserve_signature
def greet(name: str) -> str:
return f"Hello, {name}!"
print(inspect.signature(greet)) # Output: (name: str) -> str
This example shows how to use inspect.signature
to preserve a function's parameter signature, which is very useful in scenarios requiring type checking or automatic documentation generation.
Real-World Applications
Let's look at some more complex real-world application scenarios. These examples will show how decorators can play a role in actual projects:
- Cache Decorator (with Expiration Time):
import time
from functools import wraps
def cache_with_timeout(timeout=5):
def decorator(func):
cache = {}
@wraps(func)
def wrapper(*args, **kwargs):
key = str(args) + str(kwargs)
if key in cache:
result, timestamp = cache[key]
if time.time() - timestamp < timeout:
return result
result = func(*args, **kwargs)
cache[key] = (result, time.time())
return result
return wrapper
return decorator
@cache_with_timeout(timeout=10)
def expensive_operation(x, y):
time.sleep(2) # Simulate a time-consuming operation
return x + y
print(expensive_operation(1, 2)) # This will wait 2 seconds
print(expensive_operation(1, 2)) # This will return the result immediately
time.sleep(11) # Wait for the cache to expire
print(expensive_operation(1, 2)) # This will wait 2 seconds again
This example implements a cache decorator with an expiration time. It can cache the results of a function, but when the cache time exceeds the specified timeout, it will recalculate the result.
- Parameter Validation Decorator:
from functools import wraps
def validate_types(**expected_types):
def decorator(func):
@wraps(func)
def wrapper(*args, **kwargs):
for name, expected_type in expected_types.items():
if name in kwargs:
if not isinstance(kwargs[name], expected_type):
raise TypeError(f"Argument {name} must be {expected_type}")
return func(*args, **kwargs)
return wrapper
return decorator
@validate_types(x=int, y=int)
def add(x, y):
return x + y
print(add(1, 2)) # Runs correctly
try:
print(add(1, "2")) # Raises TypeError
except TypeError as e:
print(str(e))
This decorator can be used to validate the types of function arguments. If the argument types do not match the expected ones, a TypeError will be raised.
- Retry Decorator (with Backoff Strategy):
import time
import random
from functools import wraps
def retry_with_backoff(retries=3, backoff_in_seconds=1):
def decorator(func):
@wraps(func)
def wrapper(*args, **kwargs):
x = 0
while True:
try:
return func(*args, **kwargs)
except Exception as e:
if x == retries:
raise e
sleep = (backoff_in_seconds * 2 ** x +
random.uniform(0, 1))
time.sleep(sleep)
x += 1
return wrapper
return decorator
@retry_with_backoff(retries=5, backoff_in_seconds=1)
def unreliable_function():
if random.random() < 0.7:
raise Exception("Random error")
return "Success!"
print(unreliable_function())
This decorator implements a retry mechanism with an exponential backoff strategy. When a function fails, it will retry several times, with the wait time between retries gradually increasing.
- Asynchronous Decorator:
import asyncio
from functools import wraps
def async_timed():
def wrapper(func):
@wraps(func)
async def wrapped(*args, **kwargs):
print(f'starting {func.__name__}')
start = asyncio.get_event_loop().time()
try:
return await func(*args, **kwargs)
finally:
end = asyncio.get_event_loop().time() - start
print(f'{func.__name__} took {end:.4f} second(s)')
return wrapped
return wrapper
@async_timed()
async def delay(seconds):
await asyncio.sleep(seconds)
async def main():
await delay(1)
asyncio.run(main())
This decorator can be used for asynchronous functions, printing the start and end time of the function, as well as the execution time.
- Singleton Pattern Decorator:
def singleton(cls):
instances = {}
def get_instance(*args, **kwargs):
if cls not in instances:
instances[cls] = cls(*args, **kwargs)
return instances[cls]
return get_instance
@singleton
class Database:
def __init__(self):
print("Initializing database connection")
db1 = Database()
db2 = Database()
print(db1 is db2) # Output: True
This decorator implements the singleton pattern, ensuring a class has only one instance. This is useful when a globally unique object is needed (such as a database connection).
These examples demonstrate the powerful functionality of decorators in actual development. They can help us implement caching, parameter validation, error retry, performance monitoring, design patterns, etc., and can be easily applied to multiple functions or classes, greatly enhancing the reusability and maintainability of the code.
Conclusion
Alright, dear readers, our journey into Python decorators ends here. We started with the basic concepts and gradually delved into more advanced applications. You should now have a comprehensive understanding of decorators, knowing what they
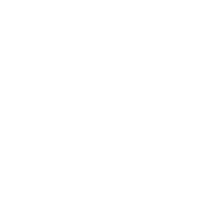
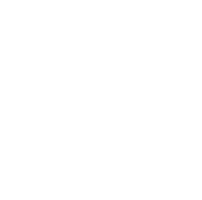