Have you ever struggled with testing complex Python projects? Have you encountered various challenges when doing integration testing? Don't worry, today we'll explore all aspects of Python integration testing together, enabling you to easily master this important skill.
Overview
Integration testing is an indispensable part of software testing. It verifies whether different modules or services work properly together, helping us discover problems that unit tests might overlook. In the Python ecosystem, we have many powerful tools and frameworks to support integration testing. Let's delve deeper together.
Why It's Important
You might ask, "I've already written unit tests, why do I need integration tests?" That's a good question. Imagine you're building a house. Unit testing is like checking the quality of each brick, while integration testing ensures that when these bricks are combined, the entire house is stable.
Integration testing can help us discover: 1. Interface issues between modules 2. Performance bottlenecks 3. Data flow anomalies 4. Environment configuration errors
These problems are often difficult to detect in unit tests.
Basic Concepts
Before diving into technical details, let's clarify a few key concepts:
- Test Fixture: Prepares and cleans up the environment for testing.
- Test Case: A group of related tests.
- Test Suite: A collection of multiple test cases.
- Test Runner: Executes tests and provides results.
Understanding these concepts will help you better organize and manage your integration tests.
Common Tools and Frameworks
The Python ecosystem provides a rich set of tools for integration testing. Let's explore some of the most commonly used and powerful tools one by one.
pytest
pytest is probably one of the most popular testing frameworks in the Python world. It's simple to use yet powerful.
Basic Usage
Using pytest is very intuitive. Suppose we have a simple function that needs testing:
def add(a, b):
return a + b
def test_add():
assert add(2, 3) == 5
To run the test, just enter in the command line:
pytest test_myapp.py
Isn't it simple? pytest will automatically discover and run all functions starting with test_
.
Advanced Features
The power of pytest lies in its rich plugin ecosystem and advanced features. For example, we can use parameterized tests:
import pytest
@pytest.mark.parametrize("a,b,expected", [
(2, 3, 5),
(0, 0, 0),
(-1, 1, 0),
])
def test_add_parametrized(a, b, expected):
assert add(a, b) == expected
This way, we can cover multiple scenarios with one test function. Very convenient, isn't it?
unittest.mock
Mocking is an important technique in integration testing. The unittest.mock library provides powerful mocking capabilities.
Suppose we have a function that depends on an external API:
import requests
def get_user_data(user_id):
response = requests.get(f"https://api.example.com/users/{user_id}")
return response.json()
We can mock the API call like this:
from unittest.mock import patch
@patch('requests.get')
def test_get_user_data(mock_get):
mock_get.return_value.json.return_value = {"name": "John", "age": 30}
result = get_user_data(1)
assert result == {"name": "John", "age": 30}
This way, we can test the function's behavior without actually calling the API. Great, right?
tox
tox is an automation tool that can run tests in multiple Python environments. It's particularly suitable for projects that need to support multiple Python versions.
A typical tox.ini file might look like this:
[tox]
envlist = py36,py37,py38
[testenv]
deps = pytest
commands = pytest
Running the tox
command will automatically run tests in Python 3.6, 3.7, and 3.8 environments. This is very useful for ensuring your code works correctly across different Python versions.
pytest-cov
Code coverage is an important metric for measuring test quality. The pytest-cov plugin can help us generate coverage reports.
After installing pytest-cov, run:
pytest --cov=myapp tests/
This will generate a detailed coverage report, helping you identify which parts of your code haven't been tested yet.
Integration Testing for Specific Frameworks
Different web frameworks may require different testing strategies. Let's look at how to write integration tests for Flask and Django applications.
Integration Testing for Flask Applications
Flask is a lightweight web framework, very suitable for rapid development. Testing Flask applications is also relatively simple.
Using pytest to Test Flask Applications
Suppose we have a simple Flask application:
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/hello')
def hello():
return jsonify({"message": "Hello, World!"})
We can test it like this:
import pytest
from myapp import app
@pytest.fixture
def client():
return app.test_client()
def test_hello(client):
response = client.get('/hello')
assert response.status_code == 200
assert response.json == {"message": "Hello, World!"}
Here, we use Flask's test_client()
to simulate HTTP requests. Convenient, isn't it?
Setting Up Test Clients and Mocking Requests
For more complex scenarios, we might need to set up some context in our tests. For example:
@pytest.fixture
def app():
app = create_app('testing')
with app.app_context():
yield app
@pytest.fixture
def client(app):
return app.test_client()
def test_create_user(client):
response = client.post('/users', json={"name": "Alice", "email": "[email protected]"})
assert response.status_code == 201
assert "id" in response.json
This way, we can test functionality that requires application context.
Integration Testing for Django Applications
As a full-stack web framework, Django provides more built-in testing tools.
Using Django's Built-in Testing Framework
Django's TestCase class is a subclass of unittest.TestCase, but with some Django-specific functionality added.
Suppose we have a simple Django view:
from django.http import JsonResponse
def hello(request):
return JsonResponse({"message": "Hello, World!"})
We can test it like this:
from django.test import TestCase, Client
class HelloViewTest(TestCase):
def setUp(self):
self.client = Client()
def test_hello(self):
response = self.client.get('/hello/')
self.assertEqual(response.status_code, 200)
self.assertEqual(response.json(), {"message": "Hello, World!"})
Application of TestCase and Client Classes
For tests that require database interaction, Django's TestCase class is particularly useful:
from django.test import TestCase
from myapp.models import User
class UserModelTest(TestCase):
def setUp(self):
User.objects.create(name="Alice", email="[email protected]")
def test_user_creation(self):
user = User.objects.get(name="Alice")
self.assertEqual(user.email, "[email protected]")
This test will run in a temporary database, not affecting your actual data. Safe, right?
Integration Testing in Docker Environments
In modern development, Docker has become a standard tool. Running integration tests in a Docker environment has many advantages, such as environment consistency and isolation.
Defining Test Environment with Dockerfile
First, we need a Dockerfile to define the test environment:
FROM python:3.8
WORKDIR /app
COPY requirements.txt .
RUN pip install -r requirements.txt
COPY . .
CMD ["pytest"]
This Dockerfile creates an image containing our application and all its dependencies.
Managing Multiple Service Dependencies with docker-compose
For applications that require multiple services (such as databases, caches, etc.), we can use docker-compose:
version: '3'
services:
app:
build: .
depends_on:
- db
db:
image: postgres:12
environment:
POSTGRES_DB: testdb
POSTGRES_PASSWORD: testpass
This way, we can run tests in a complete environment.
Configuring Network and Database Connections in Docker Containers
In a Docker environment, services communicate with each other using network names. For example, our application might need to configure the database connection like this:
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.postgresql',
'NAME': 'testdb',
'USER': 'postgres',
'PASSWORD': 'testpass',
'HOST': 'db',
'PORT': '5432',
}
}
Note that the 'HOST' here is 'db', which is the service name we defined in docker-compose.
Best Practices for Integration Testing
After discussing so many technical details, let's summarize the best practices for integration testing.
Maintaining Test Independence
Each test should be independent, not relying on the results of other tests. This makes tests more reliable and easier to debug.
For example, don't write tests like this:
def test_create_user():
# Create user...
def test_delete_user():
# Delete the user created in the previous test...
Instead, each test should set up its own required environment:
def test_create_user():
# Create user...
# Verify user creation successful...
def test_delete_user():
# Create a user
# Delete this user
# Verify user deletion successful...
Using Real Dependencies vs. Mocking
In integration testing, we usually want to use real dependencies rather than mocks. This better simulates the real environment.
However, for some external services that are difficult to control or very slow, mocking might be a better choice. The key is to weigh the pros and cons.
Ensuring Test Repeatability
Repeatability is a key characteristic of good tests. The test results should be consistent no matter how many times they are run.
Using fixed test data and avoiding dependencies on current time or random numbers all help improve test repeatability.
Automating Integration Tests in CI/CD Pipelines
Incorporating integration tests into the Continuous Integration/Continuous Deployment (CI/CD) process is a best practice in modern software development.
You can add steps to run tests in your CI/CD configuration file. For example, in GitLab CI:
test:
stage: test
script:
- pip install -r requirements.txt
- pytest
This way, tests will run automatically with every code push, helping you detect problems early.
Conclusion
Python integration testing is a broad topic, and we've only scratched the surface today. But I hope this article gives you a good start and helps you better understand and apply integration testing.
Remember, good tests not only improve code quality but also make you more confident in your code. So start writing tests, you'll find it's actually a very interesting thing to do.
Do you have any experiences or questions about Python integration testing? Feel free to share in the comments section, let's learn and grow together.
Finally, I hope this article has been helpful to you. If you found it valuable, why not share it with your colleagues and friends? Let's improve our testing skills together and write better Python code.
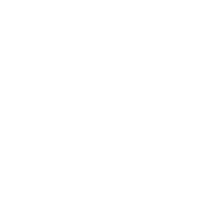
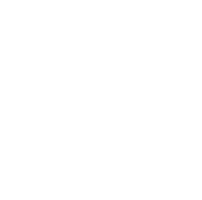