Introducing Python
Have you ever heard your friends talking about Python? Maybe you're not too familiar with programming yet, but you always feel like you should learn something to keep up with this digital age. Hey, don't worry! Today, let me take you into the magical world of Python and explore this powerful yet friendly programming language together.
Remember playing with building blocks as a kid? Python is like the Lego of the programming world. You can use it to build all sorts of amazing things, from simple calculators to complex artificial intelligence systems. As long as you have ideas, Python can help you make them a reality.
So, what's the magic of Python? First off, its syntax is incredibly concise and straightforward. You don't need to write a bunch of verbose code like in other languages. Check out this example:
print("Hello, World!")
Just like that, you've completed your first Python program! Isn't that magical?
The Magic of Variables
In the world of Python, variables are like magic pockets that can store all sorts of things. You can put numbers, text, and even more complex data in them.
age = 25
name = "Alice"
is_student = True
print(f"My name is {name}, I'm {age} years old, and I'm a student: {is_student}")
See, we just created three variables that store age, name, and student status information. Then, we used a formatted string to print them all out. Doesn't it feel like you're starting to master some magic already?
I find the concept of variables particularly interesting. You can think of it as labeling different boxes and putting things inside them. When you need to use them later, you just need to remember the label, not what's specifically inside. This is incredibly useful when dealing with large amounts of data.
Conditional Statements
In life, we often need to make choices. Python uses if statements for conditional logic, just like we do.
temperature = 30
if temperature > 25:
print("It's really hot today!")
elif temperature < 10:
print("It's a bit chilly today!")
else:
print("The weather is just right!")
This code is like a little assistant that gives you weather advice based on the conditions. Doesn't it resemble how we think? If... then..., else if... then..., finally... Python is just that intuitive!
Loop Spells
Sometimes, we need to repeat certain actions. In Python, we can use loops to achieve this.
for i in range(5):
print(f"This is the {i+1}th time printing")
count = 0
while count < 5:
print(f"While loop: This is the {count+1}th time printing")
count += 1
See that? We used a for loop and a while loop to print 5 times each. For loops are better when you know how many times to repeat, while while loops are better when you're not sure how many times, but you know when to stop.
Personally, I particularly like for loops because they remind me of the hopscotch game I played as a kid. You need to hop one step at a time until you've completed all the squares. Python's for loop works the same way, executing one step at a time until all tasks are completed.
Function Magic
Functions are like your own custom magic spells. You define what they do, and then you can use that magic with just one command.
def greet(name):
return f"Hello, {name}! Welcome to the magical world of Python!"
message = greet("Alice")
print(message)
Here, we defined a function called greet that generates a welcome message based on the input name. Doesn't it feel like your magic is getting stronger?
I find functions to be one of the most interesting parts of Python. It's like creating new spells in your magic book. You can design all sorts of functions based on your needs, making programming incredibly flexible and fun.
Lists and Dictionaries
In the magical world of Python, lists and dictionaries are two incredibly powerful data structures.
Lists are like ordered boxes where you can put all sorts of things:
fruits = ["Apple", "Banana", "Orange"]
fruits.append("Grape")
print(fruits)
Dictionaries, on the other hand, are like magic dictionaries where each word (key) corresponds to its definition (value):
person = {
"name": "Bob",
"age": 30,
"job": "Wizard"
}
print(f"{person['name']} is a {person['age']} year old {person['job']}")
Did you notice that lists use square brackets [], while dictionaries use curly braces {}? These are some of Python's little details, and remembering them will make your coding journey smoother.
Personally, I find lists and dictionaries to be the most useful data structures in Python. Lists are like our daily shopping lists, while dictionaries are like our address books. Can you imagine programming without using these two structures? They're simply indispensable!
Module Magic
Python's power also lies in its rich library of modules. Modules are like pre-prepared magic scrolls; you just need to summon them, and you can use the magic inside.
import random
random_number = random.randint(1, 100)
print(f"Random number: {random_number}")
import datetime
current_time = datetime.datetime.now()
print(f"The current time is: {current_time}")
See, with just a few lines of code, we implemented random number generation and getting the current time. That's the magic of modules!
I remember when I first started learning Python, discovering modules was a pleasant surprise. It was like opening a treasure trove filled with ready-to-use tools. This greatly accelerated my learning progress and development efficiency.
File Operations
In Python, reading and writing files is like casting a save and summon spell.
with open("magic_spell.txt", "w") as file:
file.write("Abracadabra!")
with open("magic_spell.txt", "r") as file:
content = file.read()
print(f"Magic spell read: {content}")
This code first creates a file and writes some text to it, then reads the content of that file. Doesn't it feel like you've mastered the power of information?
I find file operations to be an incredibly useful feature in Python. It allows us to persistently store data, which is especially useful when dealing with large amounts of information. You can think of it as adding a "save" feature to your magical world.
Exception Handling
Even the most powerful wizards can make mistakes, so we need to learn how to handle exceptions.
try:
number = int(input("Please enter a number: "))
result = 10 / number
print(f"10 divided by {number} is: {result}")
except ValueError:
print("Invalid input, please make sure you entered a number.")
except ZeroDivisionError:
print("Cannot divide by zero!")
except Exception as e:
print(f"An error occurred: {e}")
else:
print("Calculation completed successfully!")
finally:
print("This line will always be printed, no matter what.")
This code demonstrates how to gracefully handle various possible errors. It's like adding a protective shield to your magic, making your program more robust.
Personally, I believe exception handling is an important distinction between novices and experts. It reflects a programmer's ability to anticipate and handle different situations. By mastering exception handling, your programs can run stably in complex environments.
Object-Oriented Programming
Object-Oriented Programming (OOP) is a crucial concept in Python. It allows us to create our own "magical creatures."
class Wizard:
def __init__(self, name, power):
self.name = name
self.power = power
def cast_spell(self):
print(f"{self.name} cast a spell with power {self.power}!")
harry = Wizard("Harry", 100)
harry.cast_spell()
In this example, we created a "Wizard" class and gave it a name and power. Then, we can create specific wizard instances and have them cast spells.
I find object-oriented programming to be one of the most interesting parts of Python. It allows us to think about and solve problems in a way that's closer to the real world. You can think of it as creating a virtual world where each object has its own attributes and behaviors.
Data Science Magic
Python is also incredibly powerful in the field of data science. Let's take a look at how to use the powerful NumPy and Pandas libraries.
import numpy as np
import pandas as pd
arr = np.array([1, 2, 3, 4, 5])
print(f"NumPy array: {arr}")
print(f"Array mean: {np.mean(arr)}")
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Paris', 'London']
}
df = pd.DataFrame(data)
print("Pandas DataFrame:")
print(df)
NumPy allows us to perform efficient numerical computations, while Pandas provides powerful data processing tools. These two libraries are widely used in data analysis, machine learning, and more.
Personally, I believe mastering these data science tools is like adding a "data wizard" title to your repertoire. They enable you to extract valuable information from vast amounts of data, which is an incredibly important skill in today's big data era.
Web Scraping
Python can also help us collect information from the internet through web scraping.
import requests
from bs4 import BeautifulSoup
url = "https://www.python.org"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
title = soup.title.string
print(f"Website title: {title}")
links = soup.find_all('a')
print(f"The website contains {len(links)} links")
This code demonstrates how to use the requests library to fetch a website's content, then use BeautifulSoup to parse the HTML and extract the information we need.
I think web scraping is like giving us a pair of "far-seeing eyes," allowing us to automatically gather information from the internet. But remember to follow relevant laws and website terms of service when using this skill.
Artificial Intelligence and Machine Learning
Python is also widely used in the fields of artificial intelligence and machine learning. Let's look at a simple machine learning example:
from sklearn import datasets
from sklearn.model_selection import train_test_split
from sklearn.neighbors import KNeighborsClassifier
from sklearn.metrics import accuracy_score
iris = datasets.load_iris()
X = iris.data
y = iris.target
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
knn = KNeighborsClassifier(n_neighbors=3)
knn.fit(X_train, y_train)
y_pred = knn.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print(f"Model accuracy: {accuracy:.2f}")
This example uses the scikit-learn library to train a simple K-Nearest Neighbors classifier to predict the species of iris flowers.
Personally, I believe machine learning is like teaching computers a new way of thinking. It enables them to learn patterns from large amounts of data and then make predictions on new situations. This ability has revolutionary applications in many fields, from image recognition to natural language processing to recommendation systems, all of which rely heavily on machine learning.
Graphical User Interfaces
Python can not only run in the command line but also create graphical user interfaces (GUIs). Let's look at an example of creating a simple GUI using the Tkinter library:
import tkinter as tk
def greet():
name = name_entry.get()
greeting.config(text=f"Hello, {name}!")
window = tk.Tk()
window.title("Python GUI Magic")
name_label = tk.Label(window, text="Enter your name:")
name_label.pack()
name_entry = tk.Entry(window)
name_entry.pack()
greet_button = tk.Button(window, text="Greet", command=greet)
greet_button.pack()
greeting = tk.Label(window, text="")
greeting.pack()
window.mainloop()
This code creates a simple window that prompts the user to enter their name, and then displays a greeting message when the button is clicked.
I think GUI programming gives Python a new form of expression. It allows us to create more intuitive and interactive applications. You can think of it as giving your magic a beautiful exterior, making it not only powerful but also more attractive to people.
Game Development
Python can even be used for game development! Let's look at an example of creating a simple game using the Pygame library:
import pygame
import random
pygame.init()
width = 800
height = 600
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption("Python Magic Ball")
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
ball_radius = 20
ball_x = width // 2
ball_y = height // 2
ball_speed_x = 5
ball_speed_y = 5
running = True
clock = pygame.time.Clock()
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# Move ball
ball_x += ball_speed_x
ball_y += ball_speed_y
# Collision detection
if ball_x <= ball_radius or ball_x >= width - ball_radius:
ball_speed_x = -ball_speed_x
if ball_y <= ball_radius or ball_y >= height - ball_radius:
ball_speed_y = -ball_speed_y
# Draw
screen.fill(BLACK)
pygame.draw.circle(screen, RED, (ball_x, ball_y), ball_radius)
pygame.display.flip()
# Control frame rate
clock.tick(60)
pygame.quit()
This simple game creates a bouncing ball on the screen.
Personally, I think game development is one of the most exciting applications of Python. It allows us to transform our programming knowledge into something playable, and that instant feedback and sense of achievement is incredibly exciting. You can think of it as using code to create a little magical world, where you're the creator and the rule-maker.
Network Programming
Python can also be used for network programming, allowing our programs to communicate over the network. Here's a simple example of a server and client:
import socket
server = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server.bind(('localhost', 9999))
server.listen(1)
print("Waiting for connection...")
client, addr = server.accept()
print(f"Connection from: {addr}")
while True:
data = client.recv(1024).decode('utf-8')
if not data:
break
print(f"Received message: {data}")
response = f"Server received your message: {data}"
client.send(response.encode('utf-8'))
client.close()
server.close()
import socket
client = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client.connect(('localhost', 9999))
while True:
message = input("Enter a message ('quit' to exit): ")
if message.lower() == 'quit':
break
client.send(message.encode('utf-8'))
response = client.recv(1024).decode('utf-8')
print(f"Server response: {response}")
client.close()
This example creates a simple server and client that can send messages to each other.
I think network programming is like giving our programs the ability to communicate remotely. It allows us to create distributed applications and enables collaboration between different computers. You can think of it as establishing a magical communication channel between different wizards, enabling them to communicate and collaborate from a distance.
Data Visualization
Python is also incredibly powerful when it comes to data visualization. Let's look at an example of creating a chart using the Matplotlib library:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 =np.cos(x)
plt.figure(figsize=(10, 6))
plt.plot(x, y1, label='sin(x)')
plt.plot(x, y2, label='cos(x)')
plt.xlabel('x')
plt.ylabel('y')
plt.title('Sin and Cos Functions')
plt.legend()
plt.grid(True)
plt.show()
This code creates a plot containing the sine and cosine functions.
Personally, I find data visualization to be one of the most captivating features of Python. It allows us to transform dull numbers into visual representations, helping us better understand the patterns and trends behind the data. You can think of it as dressing up your data in a beautiful outfit, making it more attractive and easier to understand.
Web Development
Python can also be used for web development. Here's an example of creating a simple web app using the Flask framework:
from flask import Flask, render_template, request
app = Flask(__name__)
@app.route('/')
def home():
return render_template('home.html')
@app.route('/greet', methods=['POST'])
def greet():
name = request.form['name']
return render_template('greet.html', name=name)
if __name__ == '__main__':
app.run(debug=True)
<!DOCTYPE html>
<html>
<head>
<title>Python Web Magic</title>
</head>
<body>
<h1>Welcome to the Python Web Magic World!</h1>
<form action="/greet" method="post">
<input type="text" name="name" placeholder="Enter your name">
<input type="submit" value="Greet">
</form>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<title>Python Web Magic</title>
</head>
<body>
<h1>Hello, {{ name }}!</h1>
<p>Welcome to the Python Web Magic World!</p>
<a href="/">Go back home</a>
</body>
</html>
This example creates a simple web app where users can enter their name and receive a personalized greeting.
I think web development gives Python a new form of expression. It allows us to showcase Python's powerful capabilities on the web, enabling more people to use the "magic" we create. You can think of it as creating a stage for your magic, where people from all over the world can come and appreciate and use your work.
Automated Testing
Python can also be used for automated testing, ensuring the quality of our code. Here's an example of unit testing using the unittest module:
import unittest
def add(a, b):
return a + b
class TestAddFunction(unittest.TestCase):
def test_add_positive_numbers(self):
self.assertEqual(add(1, 2), 3)
def test_add_negative_numbers(self):
self.assertEqual(add(-1, -1), -2)
def test_add_mixed_numbers(self):
self.assertEqual(add(-1, 1), 0)
def test_add_zero(self):
self.assertEqual(add(5, 0), 5)
if __name__ == '__main__':
unittest.main()
This example creates a simple addition function and then writes a few test cases to verify its correctness.
Personally, I believe automated testing is an essential means of ensuring code quality. It's like casting a protective spell over your magic, ensuring it works correctly in various situations. By writing tests, we can catch and fix issues earlier, improving the reliability of our code.
Conclusion
Well, our Python magic journey ends here. We've explored Python's basic syntax, data structures, object-oriented programming, file operations, exception handling, and touched on data science, web scraping, artificial intelligence, graphical interfaces, game development, network programming, data visualization, web development, and automated testing.
Can you feel the magic of Python? Not only is its syntax concise and easy to learn, but it also has a powerful ecosystem that can be used for almost anything. From handling daily small tasks to developing large-scale projects, Python can do it all.
Remember, learning to program is like learning magic – it requires constant practice and exploration. Don't be afraid to make mistakes; every error is an opportunity to learn. Stay curious and brave enough to try new things.
Finally, I'd like to ask you: which part of this article interested you the most? Was it the powerful analysis capabilities of data science or the magical predictions of artificial intelligence? Perhaps it was the creativity of game development or the practicality of web development? No matter which one it was, I encourage you to explore it further because each field has its unique charm and infinite possibilities.
The world of Python is vast and boundless, and your programming journey has just begun. Keep moving forward, believe in yourself, and you will eventually become an outstanding Python wizard!
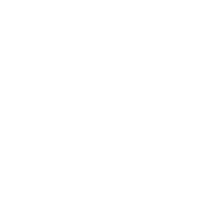
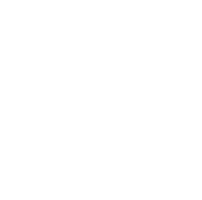