Hey, Python enthusiasts! Today we're diving into a frequently overlooked yet crucial topic - Python integration testing. Have you ever heard someone say, "My unit tests passed, but the program still has bugs"? That's exactly what we're going to discuss today. Let's delve into the mysteries of integration testing and see how it can become your secret weapon for ensuring Python project quality.
What is Integration
First, we need to understand what "integration" means. Imagine you're assembling a supercomputer. You have the CPU, memory, hard drive, and other components, each tested individually with no issues. But what happens when you put them all together? That's right, unexpected problems may arise. This is why we need integration testing.
In the Python world, integration testing is the process of checking if different modules or components can coexist harmoniously. It's not just about piecing together a few functions; it's about ensuring the entire system runs smoothly, like a precision clock with every gear perfectly meshed.
Why It's Important
You might ask, "I've already done unit testing; why do I need integration testing?" Good question! Let me give you an example.
Suppose you're developing an online shopping system. You have modules for user registration, product display, shopping cart, and payment. Each module tests fine individually, but unexpected issues may occur when a user goes from registration to completing a purchase. For instance, user information might not be correctly passed to the payment module, causing payment failure. This is something unit tests can't catch, but integration tests can.
The importance of integration testing lies in: 1. Detecting interaction issues between modules 2. Verifying the overall functionality of the system 3. Improving code quality and reliability 4. Reducing the risk of severe bugs in the production environment
I personally think of integration testing as a comprehensive health check for your code. Unit tests might only check blood pressure and heart rate, while integration tests are a full-body CT scan, uncovering potential health issues.
Types of Testing
When it comes to types of integration testing, there are mainly the following:
Incremental
This method is like building with blocks, adding modules one by one, testing each time you add one. It can be done in two ways: top-down and bottom-up.
- Top-down: Start with the main functionality, gradually adding sub-modules.
For example, when developing a blogging system, you might first test the article publication feature, then gradually add features like comments and user management.
- Bottom-up: Start with basic components, gradually building up complex features.
Again, in the blogging system example, you might first test database connections and user authentication, then test article publication and comments.
Big Bang
This method is like, well, a big bang, combining all modules at once for testing. Sounds exciting, right? But be careful, if issues arise, pinpointing the problem can be a headache.
Sandbox Testing
This method is like creating a safe playground for your code. You can test various scenarios in this controlled environment without worrying about affecting the real production environment.
I personally prefer incremental testing, especially the bottom-up approach. It allows me to gradually build confidence, ensuring each basic component is reliable before building more complex features. Although it might take more time, in the long run, it significantly reduces debugging time and effort.
Tool Selection
Speaking of tools, there are plenty of choices in the Python world. But today, I want to highlight my favorite testing framework - Pytest.
Why Pytest? Because it's simple, powerful, and flexible. It supports unit testing and is also great for integration testing. Let me show you the charm of Pytest:
import pytest
from myapp.user import User
from myapp.product import Product
from myapp.order import Order
@pytest.fixture
def user():
return User("Alice", "[email protected]")
@pytest.fixture
def product():
return Product("Laptop", 1000)
def test_user_order_integration(user, product):
order = Order(user, product)
assert order.user.name == "Alice"
assert order.product.name == "Laptop"
assert order.total_price == 1000
# Simulate order processing
order.process()
assert order.status == "Processed"
assert user.orders == [order]
See, isn't it intuitive? This test case simulates a user placing an order, checking the interaction between user, product, and order. This is a typical integration testing scenario.
Pytest's fixture
feature is particularly useful, allowing you to create reusable test data or objects. In the example above, we created user
and product
fixtures, which can be reused in multiple test cases.
Best Practices
With all this said, I'd like to share some tips I've learned from practice:
-
Maintain Test Independence: Each test should be able to run independently and not rely on the results of other tests.
-
Use Real Databases: While mock objects are handy in unit tests, in integration tests, try to use real databases or services. This can more accurately simulate the production environment.
-
Test Boundary Conditions: Don't just test normal cases; also test various exceptional conditions. For example, how should order processing react when a user's balance is insufficient?
-
Automation: Integrate integration tests into the CI/CD process, running tests automatically with every code submission.
-
Performance Considerations: Integration tests might be slower than unit tests, so pay attention to optimizing test runtime.
-
Clean Test Data: Ensure all test data is cleaned up after each test to avoid affecting subsequent tests.
I particularly want to emphasize the importance of automation. There was once a time when our team forgot to run integration tests before deploying a new version, resulting in a severe production incident. Since then, we've fully automated integration testing, making it an indispensable part of the deployment process.
Real-World Example
Let's look at a more complex real-world example. Suppose we are developing an online course platform involving user registration, course purchase, and learning progress tracking.
import pytest
from myapp.user import User
from myapp.course import Course
from myapp.enrollment import Enrollment
from myapp.progress import Progress
@pytest.fixture
def user():
return User("Bob", "[email protected]")
@pytest.fixture
def course():
return Course("Advanced Python", 299)
def test_course_enrollment_and_progress(user, course):
# Test course purchase
enrollment = Enrollment(user, course)
assert enrollment.user == user
assert enrollment.course == course
assert user.enrollments == [enrollment]
# Test learning progress
progress = Progress(enrollment)
assert progress.enrollment == enrollment
assert progress.completed_lessons == 0
# Simulate completing a lesson
progress.complete_lesson(1)
assert progress.completed_lessons == 1
assert progress.percentage == (1 / course.total_lessons) * 100
# Test course completion
for i in range(2, course.total_lessons + 1):
progress.complete_lesson(i)
assert progress.is_completed
assert user.completed_courses == [course]
def test_multiple_course_enrollments(user):
course1 = Course("Python Basics", 199)
course2 = Course("Web Development", 399)
enrollment1 = Enrollment(user, course1)
enrollment2 = Enrollment(user, course2)
assert len(user.enrollments) == 2
assert user.total_spent == 598
# Test that partially completed courses are not marked as completed
progress1 = Progress(enrollment1)
progress1.complete_lesson(1)
assert len(user.completed_courses) == 0
This test case covers multiple aspects of integration testing, such as user purchasing courses, tracking learning progress, and completing courses. It not only tests the correctness of each component but also verifies their interactions.
Through such integration tests, we can ensure: 1. Users can purchase courses correctly 2. Learning progress is accurately recorded 3. Course completion status is correctly updated 4. Users can enroll in multiple courses simultaneously 5. User's total spending is calculated correctly
See, this is the charm of integration testing. It allows us to simulate real user behavior and test the entire system's workflow.
Common Pitfalls
When conducting integration testing, there are some common pitfalls to be aware of:
-
Inconsistency between Test and Production Environments: This can lead to tests passing but issues still occurring in production. The solution is to keep the test environment as consistent with the production environment as possible, or use container technology to ensure environment consistency.
-
Neglecting Performance Testing: Integration testing should focus on performance as well as functionality. For example, in our course platform, can the system handle a large number of users purchasing courses simultaneously?
-
Insufficient Test Coverage: Testing only the normal flow is not enough; consider various boundary and exceptional cases. For instance, how should the system respond if a user tries to purchase a course that has been removed?
-
Improper Test Data Management: If test data isn't cleaned up in a timely manner, it may affect subsequent tests. It's recommended to use transactions or clean up data after each test.
-
Ignoring External Dependencies: If your system relies on external services (like payment gateways), consider how to simulate these services in tests or use sandbox environments.
I once encountered an interesting case. Our system worked fine in the test environment, but issues arose after deployment to production. After investigation, it turned out that the production database used a different character set, causing certain special characters to be processed incorrectly. This taught me the importance of keeping test and production environments consistent.
Continuous Integration
When it comes to integration testing, we must mention Continuous Integration (CI). CI is a software development practice where team members frequently integrate their work, usually at least once a day, leading to multiple integrations each day. Each integration is verified by an automated build (including compilation, release, and automated testing) to detect integration errors as quickly as possible.
In Python projects, we can use tools like Jenkins, GitLab CI, or GitHub Actions to implement continuous integration. Here's how we can create a workflow with GitHub Actions to run tests automatically:
name: Python CI
on: [push, pull_request]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: '3.x'
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r requirements.txt
- name: Run tests
run: |
pytest tests/
This workflow runs tests automatically on every code push or pull request. This way, we can catch and fix issues before they escalate.
I recall a time when our team didn't run integration tests in time, allowing a bug to linger for a long time before being discovered. Since then, we've established a strict CI process, ensuring that every code change triggers a full test suite. This not only improved our code quality but also significantly reduced the occurrence of online issues.
Conclusion and Outlook
Alright, our Python integration testing journey ends here. Let's recap the key points we've learned:
- Integration testing is crucial for verifying if different modules can work together
- It can find issues that unit testing cannot
- Pytest is a powerful framework, especially suitable for integration testing
- Automation and continuous integration are key to ensuring code quality
However, integration testing isn't a cure-all. It needs to be combined with unit testing, functional testing, and other testing methods to fully ensure software quality.
Looking ahead, with the increasing popularity of microservices architecture, the importance of integration testing will only grow. We may need more intelligent testing tools that can automatically identify system changes and generate corresponding test cases. The application of artificial intelligence in testing is also a direction worth looking forward to.
Finally, I'd like to say that testing shouldn't be a burden but a powerful tool to improve code quality and boost development confidence. I hope this article has given you a deeper understanding of Python integration testing and that you can apply it flexibly in your work.
So, do you have any experiences or questions about integration testing? Feel free to share and discuss in the comments section. Let's improve the quality of Python projects together and write more reliable, powerful code!
Practice Exercise
After learning so much, how about a little exercise to consolidate your knowledge? Imagine you're developing a simple blog system with three main modules: users, articles, and comments. Try writing some integration tests for this system. Consider the following scenarios:
- User registration and login
- User publishing an article
- Other users commenting on the article
- Article author replying to comments
How would you design these test cases? What boundary conditions need to be considered? Feel free to share your thoughts in the comments!
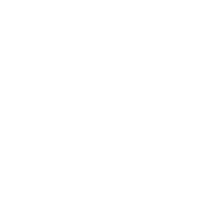
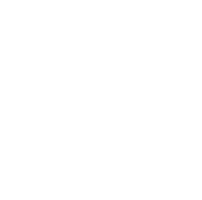