Have you ever encountered a situation where all the unit tests pass, but the program still encounters unexpected issues when running in production? If so, you might need to learn about integration testing. Today, let's dive deep into Python integration testing and see how it can help us build more reliable and robust applications.
Overview
Integration testing is a crucial part of software testing. It mainly focuses on the interactions between different modules or components, ensuring they work correctly together. Compared to unit tests, integration tests are closer to real application scenarios and can uncover issues that unit tests might miss.
In the Python ecosystem, we have many powerful tools and frameworks to support integration testing. From testing frameworks to environment management tools, to dedicated integration testing libraries, Python developers have a rich selection. Next, we'll explore these tools one by one and see how they can help us write high-quality integration tests.
Common Tools
pytest
When it comes to Python testing, pytest is a must-mention. Not only is it a powerful unit testing framework, but it also excels at integration testing. pytest's plugin ecosystem is very rich, allowing it to adapt to various complex testing scenarios.
The basic usage of pytest is straightforward. You just need to write functions starting with test_
, and pytest will automatically discover and execute these tests. For example:
def test_database_connection():
db = connect_to_database()
assert db.is_connected()
def test_api_response():
response = api.get_user_data(user_id=1)
assert response.status_code == 200
assert 'username' in response.json()
But pytest's power lies not only in simple assertion tests. Its advanced features, such as parameterized tests and fixtures, are particularly useful for integration testing.
For instance, we can use parameterized tests to test API behavior under different inputs:
import pytest
@pytest.mark.parametrize("user_id,expected_status", [
(1, 200),
(999, 404),
("invalid", 400)
])
def test_api_response_parametrized(user_id, expected_status):
response = api.get_user_data(user_id=user_id)
assert response.status_code == expected_status
This code will generate three test cases, testing the scenarios of a normal user, a non-existent user, and an invalid input. This approach can greatly reduce code duplication and improve test maintainability.
Additionally, pytest's fixtures are a very powerful feature. They can help us manage test setup and teardown, such as database connections and temporary files. For example:
import pytest
@pytest.fixture
def database():
db = connect_to_database()
yield db
db.close()
def test_database_operations(database):
user = database.create_user("Alice")
assert user.id is not None
retrieved_user = database.get_user(user.id)
assert retrieved_user.name == "Alice"
In this example, the database
fixture is responsible for creating and closing the database connection, and the test function can directly use this connection to perform operations. This approach not only simplifies the test code but also ensures proper resource management.
I personally love using pytest because it is both simple and powerful. You can start with simple assertions and gradually explore its advanced features, choosing the appropriate testing strategy based on your project's needs.
unittest.mock
When conducting integration tests, we often need to mock the behavior of certain components. This could be because these components are difficult to set up in a test environment, or because we want to control their behavior to test specific scenarios. This is where unittest.mock
comes into play.
unittest.mock
is a module in the Python standard library that provides powerful mocking capabilities. Using it, we can create mock objects, replacing parts of the system, and thereby better control the test environment.
Let's look at an example where we have a function that needs to call an external API:
import requests
def get_user_data(user_id):
response = requests.get(f"https://api.example.com/users/{user_id}")
if response.status_code == 200:
return response.json()
else:
return None
When testing this function, we might not want to actually call the external API. In this case, we can use unittest.mock
to mock the behavior of requests.get
:
from unittest.mock import patch
import pytest
def test_get_user_data():
mock_response = Mock()
mock_response.status_code = 200
mock_response.json.return_value = {"id": 1, "name": "Alice"}
with patch('requests.get', return_value=mock_response):
result = get_user_data(1)
assert result == {"id": 1, "name": "Alice"}
def test_get_user_data_not_found():
mock_response = Mock()
mock_response.status_code = 404
with patch('requests.get', return_value=mock_response):
result = get_user_data(999)
assert result is None
In this example, we use the patch
decorator (or context manager) to replace the requests.get
function. This way, we can fully control the behavior of this function and test different scenarios without making actual network requests.
unittest.mock
also allows us to create more complex mock objects. For example, we can set the attributes and methods of mock objects, and even make mock objects raise exceptions. This allows us to test various edge cases and error handling logic.
from unittest.mock import Mock, patch
def test_api_error_handling():
mock_requests = Mock()
mock_requests.get.side_effect = requests.exceptions.ConnectionError("Network error")
with patch('requests', mock_requests):
result = get_user_data(1)
assert result is None
mock_requests.get.assert_called_once_with("https://api.example.com/users/1")
In this example, we mock a network error scenario and verify that our function correctly handles this error.
I find unittest.mock
to be a very powerful tool that allows us to better control the test environment and test various complex scenarios. However, using mocks requires caution. Overusing mocks can lead to tests becoming disconnected from the actual code behavior. Therefore, when using mocks, we need to balance the controllability and realism of the tests.
Test Environment Management
tox
When conducting integration tests, we often need to run tests in different environments. This may include different Python versions, different dependency versions, etc. Manually managing these environments can be very tedious, which is where tox
comes in handy.
tox
is an automated testing tool that can help us run tests across multiple environments. Using tox
, we can easily run tests in different Python versions, ensuring our code works correctly in various environments.
To use tox
, we first need to create a tox.ini
file in the project root directory. This file defines the tox
configuration, including the environments to test, how to run the tests, etc. Here's an example of a simple tox.ini
file:
[tox]
envlist = py36,py37,py38,py39
[testenv]
deps = pytest
commands = pytest
This configuration file tells tox
to run tests in Python 3.6, 3.7, 3.8, and 3.9 environments. For each environment, it will install pytest
and then run the pytest
command.
After running the tox
command, it will automatically create virtual environments, install dependencies, and then run tests in each specified environment. This way, we can ensure our code works correctly across different Python versions.
Another powerful aspect of tox
is that it can seamlessly integrate with continuous integration (CI) systems. For example, we can use tox
in GitHub Actions:
name: Python package
on: [push]
jobs:
build:
runs-on: ubuntu-latest
strategy:
matrix:
python-version: [3.6, 3.7, 3.8, 3.9]
steps:
- uses: actions/checkout@v2
- name: Set up Python ${{ matrix.python-version }}
uses: actions/setup-python@v2
with:
python-version: ${{ matrix.python-version }}
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install tox tox-gh-actions
- name: Test with tox
run: tox
This configuration will use tox
to run tests across multiple Python versions in GitHub Actions.
I personally find tox
to be a very useful tool, especially when developing libraries that need to support multiple Python versions. It can help us avoid the "works on my machine" problem and ensure our code works correctly in various environments.
Docker
When it comes to test environment management, we can't ignore Docker. Docker is a powerful containerization platform that can help us create consistent test environments, especially suitable for complex integration tests.
Using Docker for integration testing has many advantages. First, it can ensure test environment consistency. Whether on a development machine or a CI server, we can use the same environment for testing. Second, Docker can help us simulate complex system architectures, such as interactions between multiple services.
Let's look at an example of using Docker for integration testing. Suppose we have a web application that depends on Redis and PostgreSQL. We can use docker-compose
to define this system:
version: '3'
services:
web:
build: .
ports:
- "5000:5000"
depends_on:
- db
- redis
db:
image: postgres
environment:
POSTGRES_PASSWORD: example
redis:
image: redis
This docker-compose.yml
file defines our web application, PostgreSQL database, and Redis service.
Then, we can write a test script that uses this Docker environment:
import pytest
import requests
import time
from subprocess import Popen
@pytest.fixture(scope="session")
def docker_compose_up(request):
proc = Popen(["docker-compose", "up", "-d"])
time.sleep(10) # Wait for services to start
def docker_compose_down():
Popen(["docker-compose", "down"]).wait()
request.addfinalizer(docker_compose_down)
def test_web_app(docker_compose_up):
response = requests.get("http://localhost:5000")
assert response.status_code == 200
assert "Hello, World!" in response.text
In this test, we use a pytest
fixture to start the Docker environment, run the test, and then clean up the environment. This way, we can test our application in a complete, real environment.
Docker has another significant advantage for integration testing: it can help us test different versions of dependencies. For example, we can easily test our application's behavior in different versions of a database:
version: '3'
services:
web:
build: .
ports:
- "5000:5000"
depends_on:
- db
db:
image: postgres:${POSTGRES_VERSION:-latest}
By setting the POSTGRES_VERSION
environment variable, we can easily test our application in different versions of PostgreSQL.
I personally love using Docker for integration testing. It not only helps us create consistent test environments but also simulates complex system architectures. This allows us to perform more comprehensive and realistic tests, improving our code quality and reliability.
Integration Testing for Specific Scenarios
Web Application Testing
In the Python world, web application testing is a very important topic. Whether you use Flask, Django, or FastAPI, comprehensive integration testing is key to ensuring application quality. Let's take a look at how to perform integration testing for Flask and FastAPI applications.
Flask Application Testing
Flask is a lightweight web framework that provides a test client, making it convenient for us to perform integration tests. Here's an example of a simple Flask application test:
import pytest
from flask import Flask, jsonify
@pytest.fixture
def app():
app = Flask(__name__)
@app.route('/api/users/<int:user_id>')
def get_user(user_id):
users = {1: {'name': 'Alice'}, 2: {'name': 'Bob'}}
user = users.get(user_id)
if user:
return jsonify(user)
else:
return jsonify({'error': 'User not found'}), 404
return app
@pytest.fixture
def client(app):
return app.test_client()
def test_get_user(client):
response = client.get('/api/users/1')
assert response.status_code == 200
assert response.json == {'name': 'Alice'}
def test_user_not_found(client):
response = client.get('/api/users/999')
assert response.status_code == 404
assert response.json == {'error': 'User not found'}
In this example, we use pytest
fixtures to create a Flask application and a test client. Then, we write two test cases to test the normal and error scenarios, respectively.
This approach allows us to test the entire request-response cycle, including URL routing, view function execution, and response generation. We can verify the status codes, response content, and more, ensuring our API works as expected.
FastAPI and Authentication Testing
FastAPI is a modern, fast web framework with built-in support for asynchronous programming and type hints. Testing FastAPI applications, especially those involving authentication, can be slightly more complex. Here's an example of testing with FastAPI and Keycloak for authentication:
import pytest
from fastapi.testclient import TestClient
from fastapi import FastAPI, Depends, HTTPException
from fastapi.security import OAuth2PasswordBearer
app = FastAPI()
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token")
def fake_decode_token(token):
return {"sub": "[email protected]"}
async def get_current_user(token: str = Depends(oauth2_scheme)):
user = fake_decode_token(token)
if not user:
raise HTTPException(status_code=401, detail="Invalid authentication credentials")
return user
@app.get("/users/me")
async def read_users_me(current_user: dict = Depends(get_current_user)):
return current_user
@pytest.fixture
def client():
return TestClient(app)
def test_read_main_authenticated(client):
response = client.get("/users/me", headers={"Authorization": "Bearer fake-token"})
assert response.status_code == 200
assert response.json() == {"sub": "[email protected]"}
def test_read_main_unauthenticated(client):
response = client.get("/users/me")
assert response.status_code == 401
In this example, we simulate a simple authentication system. We use TestClient
to mock HTTP requests and test the authenticated and unauthenticated scenarios.
This approach allows us to test the entire authentication flow, including token validation and user information retrieval. We can verify the behavior when authentication succeeds and fails, ensuring our API correctly handles various authentication scenarios.
I believe comprehensive integration testing for web applications is crucial. It can help us catch various edge cases and errors, improving application reliability. Especially when dealing with critical features like authentication, thorough testing can significantly increase our confidence.
Database Integration Testing
In many applications, database operations are a core functionality. Therefore, it's essential to perform comprehensive integration testing for database operations. Here, we'll explore how to use Testcontainers for database integration testing and how to manage test data.
Using Testcontainers
Testcontainers is a Java library, but it also has a Python version. It allows us to use real databases in our tests instead of mocking or in-memory databases. This makes our tests closer to the real environment.
Here's an example of using Testcontainers for PostgreSQL database testing:
import pytest
from testcontainers.postgres import PostgresContainer
import psycopg2
@pytest.fixture(scope="module")
def postgres():
postgres_container = PostgresContainer("postgres:13")
with postgres_container as postgres:
yield postgres
def test_database_operations(postgres):
conn = psycopg2.connect(
host=postgres.get_container_host_ip(),
port=postgres.get_exposed_port(PostgresContainer.PORT),
user=postgres.POSTGRES_USER,
password=postgres.POSTGRES_PASSWORD,
database=postgres.POSTGRES_DB,
)
cur = conn.cursor()
# Create table
cur.execute("CREATE TABLE test (id serial PRIMARY KEY, num integer, data varchar);")
# Insert data
cur.execute("INSERT INTO test (num, data) VALUES (%s, %s)", (100, "abc'def"))
# Query data
cur.execute("SELECT * FROM test;")
result = cur.fetchone()
assert result == (1, 100, "abc'def")
conn.commit()
cur.close()
conn.close()
In this example, we use Testcontainers to start a PostgreSQL container. Then, we connect to this database, perform a series of operations, including creating a table, inserting data, and querying data. This way, we can test our database operations in a real database environment.
Managing Test Data
When conducting database integration tests, managing test data is an important issue. We need to ensure that each test starts in a known, consistent data state. Here are a few common strategies:
- Truncate the database before each test:
@pytest.fixture(autouse=True)
def clean_database(postgres):
conn = psycopg2.connect(...)
cur = conn.cursor()
cur.execute("DROP TABLE IF EXISTS test;")
conn.commit()
cur.close()
conn.close()
- Use transaction rollback:
@pytest.fixture(autouse=True)
def transactional_test(postgres):
conn = psycopg2.connect(...)
conn.set_isolation_level(psycopg2.extensions.ISOLATION_LEVEL_AUTOCOMMIT)
cur = conn.cursor()
cur.execute("START TRANSACTION;")
yield
cur.execute("ROLLBACK;")
cur.close()
conn.close()
- Use a database migration tool:
If your project uses a database migration tool (like Alembic), you can apply all migrations before the test and then roll back after the test:
from alembic.command import upgrade, downgrade
from alembic.config import Config
@pytest.fixture(scope="session")
def apply_migrations(postgres):
alembic_cfg = Config("alembic.ini")
upgrade(alembic_cfg, "head")
yield
downgrade(alembic_cfg, "base")
Personally, I prefer using transaction rollback. This method is both fast and reliable, ensuring each test runs in a clean environment. However, the specific method to use depends on your project's requirements.
Database integration testing can be complex, but it can help us catch many issues that are difficult to detect in unit tests. By using real databases and carefully managing test data, we can greatly improve our test quality and coverage.
Test Coverage and Reporting
When conducting integration tests, it's essential to understand how much of our code is covered by our tests. This is where test coverage reports come in. In Python, we can use pytest-cov
to generate coverage reports.
pytest-cov
pytest-cov
is a pytest
plugin that can help us generate test coverage reports. Using it is straightforward; first, we need to install it:
pip install pytest-cov
Then, we can add the --cov
flag when running tests to generate coverage reports:
pytest --cov=myproject tests/
This command will run all tests in the tests/
directory and generate a coverage report for the myproject
package.
Generating Coverage Reports
pytest-cov
can generate coverage reports in various formats. By default, it will output a simple report in the console. However, we can also generate more detailed HTML reports:
pytest --cov=myproject --cov-report=html tests/
This command will create an htmlcov
directory in the current directory, containing detailed HTML coverage reports. We can open the index.html
file to view the report.
Interpreting and Improving Test Coverage
Generating coverage reports is just the first step; more importantly, we need to interpret these reports and use them to improve our tests.
-
Look at the overall coverage: This gives us a rough idea of how much of our code is covered by tests. Generally, coverage above 80% is considered decent.
-
Look at uncovered lines: The HTML report will highlight uncovered code lines. These lines are typically areas we need to focus on.
-
Focus on critical paths: Not all code is equally important. We should ensure that core functionality and critical paths have high coverage.
-
Check for boundary conditions: Low coverage might indicate that we haven't tested certain boundary conditions or error handling paths.
-
Balance cost and benefit: Pursuing 100% coverage is often impractical. We need to balance the cost and benefit of testing.
Here's an example of how to use a coverage report to improve tests:
def divide(a, b):
if b == 0:
raise ValueError("Cannot divide by zero")
return a / b
def test_divide():
assert divide(10, 2) == 5
Suppose we run a coverage report and find that the line if b == 0
is not covered. This reminds us that we need to add a test to cover the case of zero division:
import pytest
def test_divide_by_zero():
with pytest.raises(ValueError):
divide(10, 0)
Through this iterative process, we can gradually improve our test coverage and make our tests more comprehensive.
Personally, I believe test coverage is a useful metric, but it should not be the sole goal. High coverage doesn't guarantee there are no bugs, and low coverage doesn't necessarily mean poor code quality. We should treat coverage reports as a tool to help us identify potential problem areas and guide us in improving our tests. At the same time, we should also pay attention to the quality of our tests, ensuring that our tests not only cover the code but also verify the correct behavior.
Conclusion
Throughout this article, we've explored various aspects of Python integration testing in depth. From common tools and frameworks like pytest and unittest.mock, to test environment management tools like tox and Docker, to testing strategies for specific scenarios and coverage reporting, we've covered many important topics.
Integration testing is a complex but crucial topic. It helps us verify that the various parts of a system work correctly together, catching issues that unit tests might miss. By writing comprehensive integration tests, we can greatly improve the quality and reliability of our code.
However, integration testing also faces some challenges. It is typically more difficult to write and maintain than unit tests, and it also takes longer to run. Therefore, we need to strike a balance between test comprehensiveness and efficiency.
Personally, I believe a good testing strategy should include a reasonable combination of unit tests and integration tests. Unit tests can quickly validate the behavior of individual components, while integration tests can ensure that these components work together correctly.
Finally, I want to emphasize that testing should not be an afterthought; it should be an integral part of the development process. By starting to write tests early in development, we can catch and fix issues earlier, saving time and resources.
What are your thoughts or experiences with Python integration testing? Have you encountered any particularly challenging testing scenarios? Feel free to share your thoughts and experiences in the comments, and let's explore together how to write better test code.
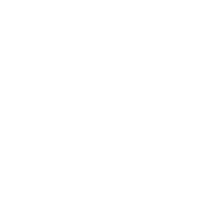
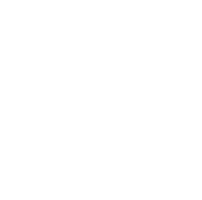