Hello, dear Python enthusiasts! Today, let's talk about integration testing in Python. This topic may sound a bit lofty, but don't worry, I'll explain it in the simplest way possible, ensuring you have an "aha" moment after reading. So, let's begin this exciting learning journey!
What is Integration
First, we need to understand what "integration" means. Imagine you're building a Lego castle. Each Lego block is like the modules in our program, and putting these blocks together to form a complete castle is "integration."
In software development, integration is combining different modules or components to form a complete system. Sounds simple, right? But in practice, it's not that easy.
The Testing Dilemma
You might ask, "Haven't we already done unit testing? Why do we need integration testing?"
Great question! Let me give you an example. Suppose you're developing an online shopping system with three modules: user login, product display, and shopping cart. Each module tests fine individually, but when combined, you find that users can't add products to the cart after logging in. This is an issue unit tests can't catch, and integration tests are designed to solve these problems.
The Charm of Integration Testing
Integration testing is like a full-body check-up for your program. It not only checks if each part is healthy but also ensures their "collaboration" is smooth. Through integration testing, we can:
- Discover interface issues between modules
- Check if data passes correctly between different modules
- Verify if the entire system functions as expected
See, integration testing is quite important, right?
Pytest: Your Handy Assistant
When it comes to integration testing in Python, we must mention the powerful testing framework, Pytest. It's like your personal assistant, helping manage and execute various tests. With Pytest, you can easily organize and run your integration tests.
Let's look at a simple example:
import pytest
from your_app import create_app, db
from your_app.models import User
@pytest.fixture
def client():
app = create_app('testing')
with app.test_client() as client:
with app.app_context():
db.create_all()
yield client
db.drop_all()
def test_user_registration(client):
response = client.post('/register', data={
'username': 'testuser',
'email': '[email protected]',
'password': 'password123'
})
assert response.status_code == 200
assert User.query.filter_by(username='testuser').first() is not None
def test_user_login(client):
# Register a user first
client.post('/register', data={
'username': 'testuser',
'email': '[email protected]',
'password': 'password123'
})
# Then try logging in
response = client.post('/login', data={
'username': 'testuser',
'password': 'password123'
})
assert response.status_code == 200
assert b'Welcome, testuser!' in response.data
In this example, we test the user registration and login features. Notice how we register a user in one test and then test login. This is the charm of integration testing—we test not only individual functions but also their interactions.
The Art of Integration Testing
Writing good integration tests isn't easy; it requires skill and experience. Here are some tips I've summarized:
-
Maintain Independence: Each test should be independent and not rely on the results of other tests.
-
Pay Attention to Order: Although tests should be independent, sometimes the order is important. For instance, you may need to test user registration before login.
-
Mock External Dependencies: If your system depends on external services (like payment systems), use mock objects to simulate these services.
-
Test Boundary Conditions: Don't only test normal cases; also test extreme cases. For example, what happens when a user enters special characters as a username?
-
Use Test Data: Create data specifically for testing rather than using production data.
Pitfall Guide
In integration testing, you might encounter common issues. Don't worry, I'll guide you through:
-
Slow Test Execution: Integration tests are usually slower than unit tests. If they're too slow, consider running full integration tests only in the CI/CD process and key tests during local development.
-
Inconsistent Test Environments: Ensure all developers and the CI/CD environment use the same test environment. Docker can solve this problem well.
-
Test Data Management: Each test should use a clean data set. Use database transactions or reset the database before each test.
-
Difficult Debugging: When integration tests fail, it can be hard to locate the issue. Use detailed logs and assertion information to help you find problems faster.
Conclusion
Well, my friend, our journey into Python integration testing ends here. Remember, integration testing is like a full-body check-up for your program; it can help you discover issues that unit tests might overlook. Although writing good integration tests requires skill, with practice, you'll soon master this art.
Are you ready to start your integration testing journey? Give it a try, and you'll discover a whole new world of testing!
By the way, if you encounter any issues in practice or have insights to share, feel free to let me know. Learning is a continuous process, and together we progress!
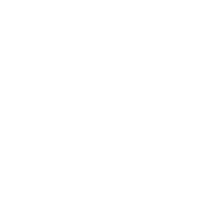
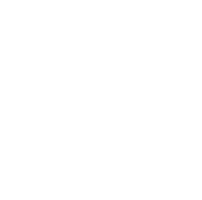