Hey, Python enthusiasts! Today, let's talk about a very important but often overlooked topic - integration testing. Have you ever heard someone say "The unit tests look good, but what about integration tests?" or "How come this bug wasn't caught in integration testing?" If you find these questions confusing, then this article is for you!
Overview
Integration testing, as the name suggests, is about testing the interaction between multiple components or modules. How does it differ from unit testing? Simply put, unit testing focuses on the behavior of individual functions or classes, while integration testing simulates real environments and tests how multiple components work together.
You might ask, "Why do we need integration testing? Isn't unit testing enough?" Good question! Let me give you an example to illustrate.
Imagine you're making a cake. Unit testing is like separately testing the quality of flour, eggs, and sugar, while integration testing is mixing these ingredients together, putting them in the oven, and seeing if you can bake a delicious cake. The individual ingredients might be fine, but when mixed together, unexpected results could occur.
This is where the importance of integration testing lies - it can uncover issues that are difficult to find in unit tests, such as mismatched interfaces between components, abnormal data flows, etc. Especially in modern complex software systems, the role of integration testing becomes even more prominent.
Common Testing Frameworks
When it comes to Python testing frameworks, we must mention the three giants: pytest, unittest, and doctest. Each has its own characteristics and is suitable for different scenarios. Let's take a look at them one by one.
pytest
pytest is my favorite testing framework and one of the most popular choices in the Python community. Why? Because it's simple to use, powerful, and has a rich plugin ecosystem.
The basic usage is very simple. You just need to write a function starting with test_
and use assert
statements to verify results:
def test_addition():
assert 1 + 1 == 2
Running tests is also easy, just type pytest
in the command line.
But the power of pytest is not just in its basic usage. It has many advanced features. For example, its parameterized testing feature is very useful:
import pytest
@pytest.mark.parametrize("input,expected", [
("hello", 5),
("world", 5),
("pytest", 6)
])
def test_string_length(input, expected):
assert len(input) == expected
This way, you can test multiple inputs with one test function. Isn't that convenient?
Moreover, pytest's fixture feature is also a highlight. It can help you manage setup and teardown operations for tests, such as database connections, temporary file creation, etc. We'll discuss this in detail later.
unittest
unittest is a testing framework that comes with the Python standard library. Its design is inspired by Java's JUnit. If you have a Java background, you might prefer unittest's style.
With unittest, you need to create a class that inherits from unittest.TestCase
, and then define methods starting with test_
in the class:
import unittest
class TestStringMethods(unittest.TestCase):
def test_upper(self):
self.assertEqual('foo'.upper(), 'FOO')
def test_isupper(self):
self.assertTrue('FOO'.isupper())
self.assertFalse('Foo'.isupper())
if __name__ == '__main__':
unittest.main()
The advantage of unittest is that it provides a rich set of assertion methods, such as assertEqual
, assertTrue
, etc., which can provide more detailed error information when tests fail.
In addition, unittest also provides setUp
and tearDown
methods for setup and teardown operations before and after tests. This is particularly useful in integration testing, as you may need to prepare the environment before testing and clean up after testing.
doctest
doctest is a very unique testing tool in Python. It allows you to write test cases in the docstring of a function. The advantage of this approach is that test cases are documentation, and documentation is test cases, ensuring that your documentation always stays consistent with your code.
Using doctest is very simple:
def add(a, b):
"""
>>> add(1, 2)
3
>>> add(-1, 1)
0
"""
return a + b
if __name__ == "__main__":
import doctest
doctest.testmod()
In this example, we wrote two test cases in the docstring of the add
function. When you run this script, doctest will automatically execute these tests.
The advantage of doctest is that it can keep your documentation up-to-date, because if code changes cause tests to fail, you'll immediately know that you need to update the documentation. However, its disadvantage is that it may not be suitable for complex testing scenarios.
Auxiliary Tools and Libraries
In addition to testing frameworks, there are some tools and libraries that can help us better perform integration testing. Let's look at two important tools: the mock library and tox.
mock library
When doing integration testing, we often need to simulate external dependencies. For example, if your code needs to call an external API, you might not want to actually call this API every time you test. This is where the mock library comes in handy.
In Python 3.3 and later versions, the mock library has been integrated into the unittest.mock module of the standard library. Using mock allows you to easily simulate the behavior of objects and functions:
from unittest.mock import Mock
mock_api_call = Mock()
mock_api_call.return_value = {"status": "success", "data": [1, 2, 3]}
result = mock_api_call()
print(result) # Output: {"status": "success", "data": [1, 2, 3]}
mock_api_call.assert_called_once()
In this example, we created a mock object to simulate an API call. We can specify the return value of this mock object, then use it in our test. Finally, we can verify if this mock object was called.
The power of the mock library lies in its ability to let you precisely control the environment of the code being tested, thus enabling more reliable and repeatable testing.
tox
When your project needs to be tested in multiple Python versions or multiple environments, tox is a very useful tool. It can help you automate the testing process, ensuring that your code works properly in different environments.
Using tox is simple, you just need to create a tox.ini
file in the root directory of your project:
[tox]
envlist = py36,py37,py38
[testenv]
deps = pytest
commands = pytest
This configuration file tells tox to run tests in Python 3.6, 3.7, and 3.8 environments, using pytest as the testing framework.
Then, you just need to run tox
in the command line, and it will automatically create virtual environments, install dependencies, and run tests for you. This way, you can ensure that your code works properly in different Python versions.
Another advantage of tox is that it can be easily integrated into CI/CD processes. For example, you can use tox in GitHub Actions to automate your testing process.
Integration Testing for Specific Frameworks
Different web frameworks may require different integration testing methods. Let's look at how to perform integration testing in FastAPI, Flask, and Django.
FastAPI
FastAPI is a modern, fast (high-performance) web framework for building APIs. Its asynchronous features make testing slightly more complex, but still manageable.
Here's an example of testing a FastAPI application using pytest:
from fastapi.testclient import TestClient
from your_app import app
client = TestClient(app)
def test_read_main():
response = client.get("/")
assert response.status_code == 200
assert response.json() == {"message": "Hello World"}
In this example, we use the TestClient
provided by FastAPI to simulate HTTP requests. This allows us to interact with our application like a real client.
If your FastAPI application uses Keycloak for authentication, you might need to simulate Keycloak's behavior. Here's a simple example:
import pytest
from httpx import AsyncClient
from your_app import app
@pytest.mark.asyncio
async def test_protected_route():
async with AsyncClient(app=app, base_url="http://test") as ac:
# Simulate Keycloak authentication
response = await ac.post("/auth", json={"username": "test", "password": "test"})
token = response.json()["access_token"]
# Access protected route using token
response = await ac.get("/protected", headers={"Authorization": f"Bearer {token}"})
assert response.status_code == 200
This example shows how to simulate the Keycloak authentication process and use the obtained token to access a protected route.
Flask
Flask is a lightweight web framework, and its testing is relatively simple. Flask provides a test client that can be used to simulate requests.
Here's an example of integration testing using pytest and Flask-Testing:
import pytest
from flask_testing import TestCase
from your_app import create_app, db
class TestWebApp(TestCase):
def create_app(self):
app = create_app('testing')
return app
def setUp(self):
db.create_all()
def tearDown(self):
db.session.remove()
db.drop_all()
def test_home_page(self):
response = self.client.get('/')
self.assert200(response)
self.assert_template_used('home.html')
def test_create_user(self):
response = self.client.post('/users', data=dict(
username='test_user',
email='[email protected]'
), follow_redirects=True)
self.assert200(response)
self.assertIn(b'User created successfully', response.data)
In this example, we created a test class that inherits from TestCase
. The create_app
method is used to create a Flask application for testing, and the setUp
and tearDown
methods are used to set up and clean up the database before and after each test. Then we defined two test methods to test the home page and user creation functionality.
Django
Django is a full-stack web framework that provides rich testing tools. We can use the pytest-django plugin to combine pytest and Django's testing functionality.
Here's an example of integration testing using pytest-django:
import pytest
from django.urls import reverse
from your_app.models import User
@pytest.mark.django_db
def test_user_creation(client):
response = client.post(reverse('create_user'), {
'username': 'testuser',
'email': '[email protected]',
'password': 'testpass123'
})
assert response.status_code == 302 # Redirect status code
assert User.objects.filter(username='testuser').exists()
@pytest.mark.django_db
def test_user_login(client):
User.objects.create_user('testuser', '[email protected]', 'testpass123')
response = client.post(reverse('login'), {
'username': 'testuser',
'password': 'testpass123'
})
assert response.status_code == 302 # Redirect status code
assert '_auth_user_id' in client.session
In this example, we use the @pytest.mark.django_db
decorator to tell pytest that this test needs to access the database. We tested the user creation and login functionality, verifying the HTTP response status code and changes in the database or session.
Integration Testing with Docker
In modern microservice architectures, our applications may depend on multiple external services. In this case, using Docker for integration testing becomes very useful. Docker allows us to run our application and its dependencies in isolated environments, enabling more realistic integration testing.
Using docker-compose
docker-compose is a tool for defining and running multi-container Docker applications. We can use it to set up our test environment.
Suppose we have a Python application that depends on Redis. We can create a docker-compose.yml
file:
version: '3'
services:
app:
build: .
depends_on:
- redis
redis:
image: redis:alpine
Then, we can use this Docker environment in our test code:
import pytest
import docker
@pytest.fixture(scope="session")
def docker_compose_file(pytestconfig):
return pytestconfig.rootdir / "docker-compose.yml"
@pytest.fixture(scope="session")
def docker_compose_project(docker_compose_file):
client = docker.from_env()
project = docker.compose.project.from_config(
project_dir=docker_compose_file.parent,
config_files=[docker_compose_file.name]
)
project.up()
yield project
project.down(remove_image_type=False, include_volumes=True)
def test_redis_connection(docker_compose_project):
redis_host = docker_compose_project.containers()[1].attrs['NetworkSettings']['Networks']['bridge']['IPAddress']
# Use redis_host to connect to Redis and perform tests
...
In this example, we use pytest's fixture feature to manage the lifecycle of the Docker environment. In the test function, we can get the IP address of the Redis container and then use this address for testing.
Using the pytest-docker plugin
pytest-docker is a very useful pytest plugin that simplifies the process of using Docker in tests. With this plugin, we can more easily manage the lifecycle of Docker containers.
Here's an example using pytest-docker:
import pytest
import redis
@pytest.fixture(scope="session")
def docker_compose_file(pytestconfig):
return pytestconfig.rootdir / "docker-compose.yml"
@pytest.fixture(scope="session")
def redis_service(docker_ip, docker_services):
"""Ensure that Redis service is up and responsive."""
port = docker_services.port_for("redis", 6379)
url = f"redis://{docker_ip}:{port}"
docker_services.wait_until_responsive(
timeout=30.0, pause=0.1, check=lambda: is_responsive(url)
)
return url
def is_responsive(url):
try:
client = redis.Redis.from_url(url)
return client.ping()
except redis.exceptions.ConnectionError:
return False
def test_redis_connection(redis_service):
client = redis.Redis.from_url(redis_service)
assert client.ping()
In this example, we defined a redis_service
fixture that ensures the Redis service is started and responsive. Then in our test function, we can directly use this fixture to get the Redis connection URL.
Test Data Management
When performing integration testing, managing test data is an important issue. We need to ensure that each test has a consistent initial state, while also being able to conveniently create data needed for various test scenarios.
Using fixtures
pytest's fixture feature is a powerful tool for managing test data. It allows us to define reusable test data and resources.
Here's an example of using fixtures to manage test data:
import pytest
from your_app.models import User
@pytest.fixture
def sample_user(db):
user = User.objects.create(
username='testuser',
email='[email protected]'
)
yield user
user.delete()
def test_user_profile(client, sample_user):
response = client.get(f'/users/{sample_user.id}/')
assert response.status_code == 200
assert sample_user.username in response.content.decode()
In this example, we defined a sample_user
fixture that creates a user before each test and deletes the user after the test. This way, we can ensure that each test has a clean initial state.
Using the factory_boy library
For more complex data models, we can use the factory_boy library to create test data. factory_boy allows us to define factory classes that can conveniently create complex objects.
Here's an example using factory_boy:
import factory
from your_app.models import User, Post
class UserFactory(factory.django.DjangoModelFactory):
class Meta:
model = User
username = factory.Sequence(lambda n: f'user{n}')
email = factory.LazyAttribute(lambda obj: f'{obj.username}@example.com')
class PostFactory(factory.django.DjangoModelFactory):
class Meta:
model = Post
title = factory.Faker('sentence')
content = factory.Faker('paragraph')
author = factory.SubFactory(UserFactory)
def test_post_list(client):
PostFactory.create_batch(5)
response = client.get('/posts/')
assert response.status_code == 200
assert len(response.json()) == 5
In this example, we defined two factory classes, UserFactory
and PostFactory
. These factory classes can conveniently create user and post objects. In the test function, we use PostFactory.create_batch(5)
to create 5 posts, then test whether the post list page correctly displays these posts.
The advantage of using factory_boy is that it can help us automatically generate reasonable test data, while also making it easy to customize this data. This makes it easier to create various test scenarios.
Conclusion
Alright, friends, our journey into Python integration testing comes to an end here. We started from the concept of integration testing, introduced common testing frameworks, auxiliary tools, and methods for performing integration testing in different web frameworks. We also discussed how to use Docker for more realistic integration testing, and how to manage test data.
Remember, although integration testing is more complex than unit testing, it can help us uncover issues that are difficult to find in unit tests. In real projects, both unit tests and integration tests are indispensable.
What do you think is the most challenging part of integration testing? Is it simulating external dependencies? Or managing test data? Feel free to share your thoughts and experiences in the comments!
Finally, I want to say that testing is not just about finding bugs, but also a design tool. By writing tests, we can think about our code from the user's perspective, which often helps us improve code design. So, let's embrace testing and write better code together!
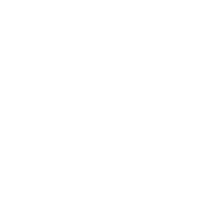
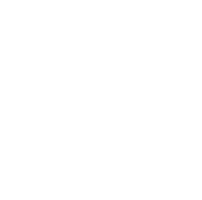