Hello, Python enthusiasts! Today we're going to discuss the fascinating and practical topic of Python web scraping. As a Python blogger, I've always been passionate about web scraping technology. It not only allows us to extract valuable information from the vast internet but also helps automate many tedious data collection tasks. Are you ready to explore the mysteries of Python web scraping with me? Let's begin this exciting learning journey!
Demystifying
What is web scraping? Simply put, it's a way to automatically obtain data we need from web pages through programming. Imagine having to manually copy and paste information from hundreds or thousands of web pages - what a tedious task that would be! With web scraping, we can write a Python script to easily complete this task. Isn't that amazing?
The basic steps of web scraping are actually quite simple:
- Send requests to get webpage content
- Parse HTML to extract needed data
- Store data for later use
Sounds easy, right? But to truly master this technology, we need to dive into some details. Don't worry, I'll guide you step by step through the mysteries of web scraping.
Applications
You might wonder, what's web scraping actually useful for? In fact, its applications are very broad. Let me give you some examples:
-
Data Analysis: Say you want to analyze price trends for certain products on an e-commerce platform. Through web scraping, you can easily obtain large amounts of price data for statistical analysis.
-
Market Research: If you want to learn about competitors' product information, web scraping can help you quickly collect relevant data.
-
Public Opinion Monitoring: By scraping comments from social media, you can stay informed about users' views on your products or services.
-
Academic Research: Many studies require large amounts of web data, and web scraping can greatly improve data collection efficiency.
I remember once writing a small Python program to scrape nearly ten thousand Python learning discussions from a forum. By analyzing this data, I discovered many interesting learning patterns and common issues. This not only helped me improve my teaching methods but also provided valuable writing material for my blog. You see, the charm of web scraping lies in this - it allows us to discover new insights from massive amounts of data!
Toolbox
When it comes to Python web scraping, we must mention some powerful tool libraries. These libraries are like our capable assistants, greatly simplifying the scraping process. Let me introduce several that I use most frequently:
-
Requests: This is a powerful tool for sending HTTP requests. Its API is designed very elegantly and is simple to use. Every time I use it, I marvel at Python's philosophy - simplicity is beauty, perfectly embodied in this library!
-
Beautiful Soup: A masterpiece for parsing HTML. It can easily handle various complex HTML structures, making data extraction effortless. I often feel that with Beautiful Soup, HTML becomes transparent, as if under a magic spell.
-
Scrapy: This is an all-in-one crawler framework. If you need to perform large-scale web scraping, Scrapy is definitely the top choice. It provides a complete set of tools, from URL management to data storage.
-
Selenium: A great helper for handling dynamic web pages. Some webpage content is dynamically loaded through JavaScript, which ordinary scraping methods might not be able to obtain. This is where Selenium comes in handy. It can simulate browser behavior, helping you easily handle various complex situations.
My favorite is still Beautiful Soup. I remember when I first used it, I felt like I had found a long-lost treasure! Tasks that previously required complex regular expressions could be done with just a few lines of code using Beautiful Soup. It improved my scraping efficiency by more than an order of magnitude.
Did you know? These tools have interesting names. Requests is straightforward, Beautiful Soup makes one think of delicious food, Scrapy vividly describes the scraping process, and Selenium is a chemical element. It seems programmers can be quite creative when naming things!
Practical Application
After all this theory, are you eager to try it yourself? Let's write a simple crawler program together! We'll take scraping article titles from a tech blog as an example.
import requests
from bs4 import BeautifulSoup
url = 'https://techblog.example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
titles = soup.find_all('h2', class_='post-title')
for title in titles:
print(title.text.strip())
This code looks simple, right? But it already contains the core steps of web scraping. Let me explain:
-
First, we use requests.get() to send a GET request and obtain the target webpage content.
-
Then, we parse the HTML using Beautiful Soup. 'html.parser' is Python's built-in HTML parser, fast and convenient to use.
-
Next, we use the find_all() method to find all h2 tags with class='post-title'. This assumes article titles are in such tags.
-
Finally, we iterate through the found titles and print them. The strip() method is used to remove any extra whitespace characters.
Look, in just a few lines of code, we've completed a simple web scraping task! Isn't it magical?
Of course, actual webpage structures might be more complex, and we might need more processing steps. But the basic approach is the same: send requests, parse HTML, extract data.
I remember the first time I successfully ran similar code, watching the data continuously print on the screen - that sense of achievement was indescribable! Try it yourself, and I believe you'll feel the same way.
Precautions
At this point, I must remind everyone that while web scraping is powerful, there are some issues to be mindful of:
-
Comply with website terms of use: Some websites explicitly prohibit crawlers, and we must respect their rules.
-
Control request frequency: Too frequent requests might burden the server or even be seen as an attack. Be like a polite guest, don't disturb the host.
-
Set appropriate User-Agent: Many websites check request headers and will refuse access if they detect a crawler. So we need to "disguise" ourselves as normal browsers.
-
Handle exceptions: Network environments are complex, and our programs need to gracefully handle various potential errors.
-
Legal use of data: Scraped data might involve copyright issues, requiring special attention when using it.
I once had my IP blocked by a website for several days because I ignored request frequency control. During that time, I deeply understood the principle of "haste makes waste." So, everyone must be cautious in practice, both improving efficiency and respecting others.
Future Outlook
Web scraping technology continues to evolve, with many future possibilities:
-
Application of Artificial Intelligence: Using machine learning algorithms, we might develop smarter crawlers that can automatically identify and extract valuable information.
-
Distributed Crawlers: As data volume increases, single-machine crawlers might become inadequate. Distributed systems will become inevitable for large-scale crawling.
-
Anti-scraping Technology Upgrades: Website anti-scraping measures will become increasingly complex, forcing us to continuously improve our technical skills.
-
Legal Framework Improvement: As web scraping technology becomes more widespread, relevant laws and regulations will gradually improve, and we need to stay informed about these developments.
What are your thoughts about the future of web scraping? Feel free to share your views in the comments!
Summary
Well, our Python web scraping journey comes to a temporary end. We've learned what web scraping is, its applications, common tools, how to write a simple crawler program, and important considerations.
Remember, web scraping isn't just a technology, it's also an art. It requires continuous learning and practice, exercising creativity while following rules to mine the value of data.
If you're interested in web scraping, try starting with some small projects. For example, you could try scraping headlines from your favorite news website or collecting the most discussed topics from a forum. Through practice, you'll discover the joy of web scraping and encounter various challenges. But don't worry, remember to come back and review this article, and feel free to ask questions in the comments.
Finally, I want to say that learning technology is a gradual process. Don't rush, and don't be intimidated by difficulties. Maintain your curiosity and enthusiasm for learning, and you'll surely go far on your Python web scraping journey.
So, are you ready to begin your web scraping journey? Let's explore this ocean of data together!
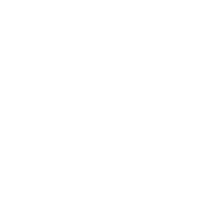
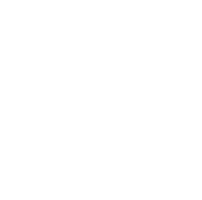