Opening Message
Have you ever wondered how massive amounts of web data are automatically collected? Or have you thought about how to use a program to help you complete those annoying repetitive data collection tasks? Today, let me share the mysteries of Python web scraping with you and see how it helps us solve these problems.
Basic Foundation
Before we start writing scrapers, you need to understand some basic concepts. Simply put, a web scraper is a program that simulates human browsing behavior to automatically retrieve web information. Just like you access web pages with a browser, a scraper also needs to send requests, receive responses, and then extract the required information.
I remember when I first encountered web scraping, I was deeply fascinated by its magic. Imagine that a simple piece of Python code can accomplish hours of manual data collection work; such an efficiency boost is truly exciting.
Tool Selection
When it comes to tool selection, I personally recommend starting with the Requests library. Why? Because its syntax is intuitive and its concepts are clear, making it particularly suitable for beginners. Here's a simple example:
import requests
import time
def get_webpage(url):
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36'
}
try:
response = requests.get(url, headers=headers)
response.raise_for_status()
return response.text
except requests.RequestException as e:
print(f"An error occurred with the request: {e}")
return None
time.sleep(1)
Do you need an explanation for this code?
Data Parsing
Data parsing is the most critical stage in web scraping. We often use BeautifulSoup to accomplish this task. It's like a smart scalpel that can accurately locate and extract the data we need. Check out this practical example:
from bs4 import BeautifulSoup
import pandas as pd
def parse_content(html_content):
if not html_content:
return []
soup = BeautifulSoup(html_content, 'html.parser')
data_list = []
# Suppose we want to extract all article titles and links
articles = soup.find_all('article')
for article in articles:
title = article.find('h2').text.strip() if article.find('h2') else 'No Title'
link = article.find('a')['href'] if article.find('a') else ''
data_list.append({'title': title, 'link': link})
return data_list
df = pd.DataFrame(data_list)
Do you need an explanation for this code?
Advanced Techniques
As you become more familiar with web scraping, you'll find that many websites use dynamic loading technology, which is when Selenium comes into play. I think Selenium is like giving scrapers a pair of eyes, allowing them to operate the browser like a human.
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
def scrape_dynamic_content(url):
driver = webdriver.Chrome()
try:
driver.get(url)
# Wait for specific element to load
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.CLASS_NAME, "target-class"))
)
# Get dynamically loaded content
content = element.text
return content
finally:
driver.quit()
Do you need an explanation for this code?
Practical Experience
In actual projects, I find that the most important thing is not to write complex code, but to consider handling various exceptions. Here, I share a template for exception handling that I often use:
import logging
from requests.exceptions import RequestException
from bs4 import BeautifulSoup
import time
class WebScraper:
def __init__(self):
self.session = requests.Session()
self.setup_logging()
def setup_logging(self):
logging.basicConfig(
level=logging.INFO,
format='%(asctime)s - %(levelname)s - %(message)s',
filename='scraper.log'
)
def safe_request(self, url, retries=3):
for i in range(retries):
try:
response = self.session.get(url, timeout=10)
response.raise_for_status()
return response
except RequestException as e:
logging.error(f"Request failed {url}: {str(e)}")
if i == retries - 1:
raise
time.sleep(2 ** i) # Exponential backoff
Do you need an explanation for this code?
Experience Summary
Through years of web scraping development experience, I have summarized a few important suggestions:
-
Web scraping development should be gradual, starting from simple static web pages and gradually transitioning to complex dynamic web pages.
-
Always pay attention to the website's robots.txt rules and adhere to its access standards. I have encountered being IP banned due to excessive scraping frequency.
-
Consider multiple solutions for data storage; don't put all your eggs in one basket. For example, use both CSV files and databases for storage.
-
The code should have a good exception handling mechanism to ensure the stability of the scraper.
Conclusion and Outlook
Web scraping technology is constantly evolving, and new challenges and opportunities will arise in the future. With the development of artificial intelligence technology, in what direction do you think web scraping technology will advance? Feel free to discuss your thoughts with me in the comments section.
Remember, learning web scraping is not an overnight process; it requires continuous practice and summary. I hope this article can give you some inspiration and help you avoid some detours on the web scraping path. If you have any questions, feel free to leave a comment for discussion.
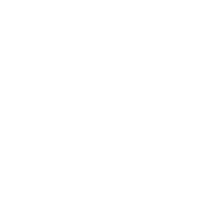